把Android原生的View渲染到OpenGL Texture
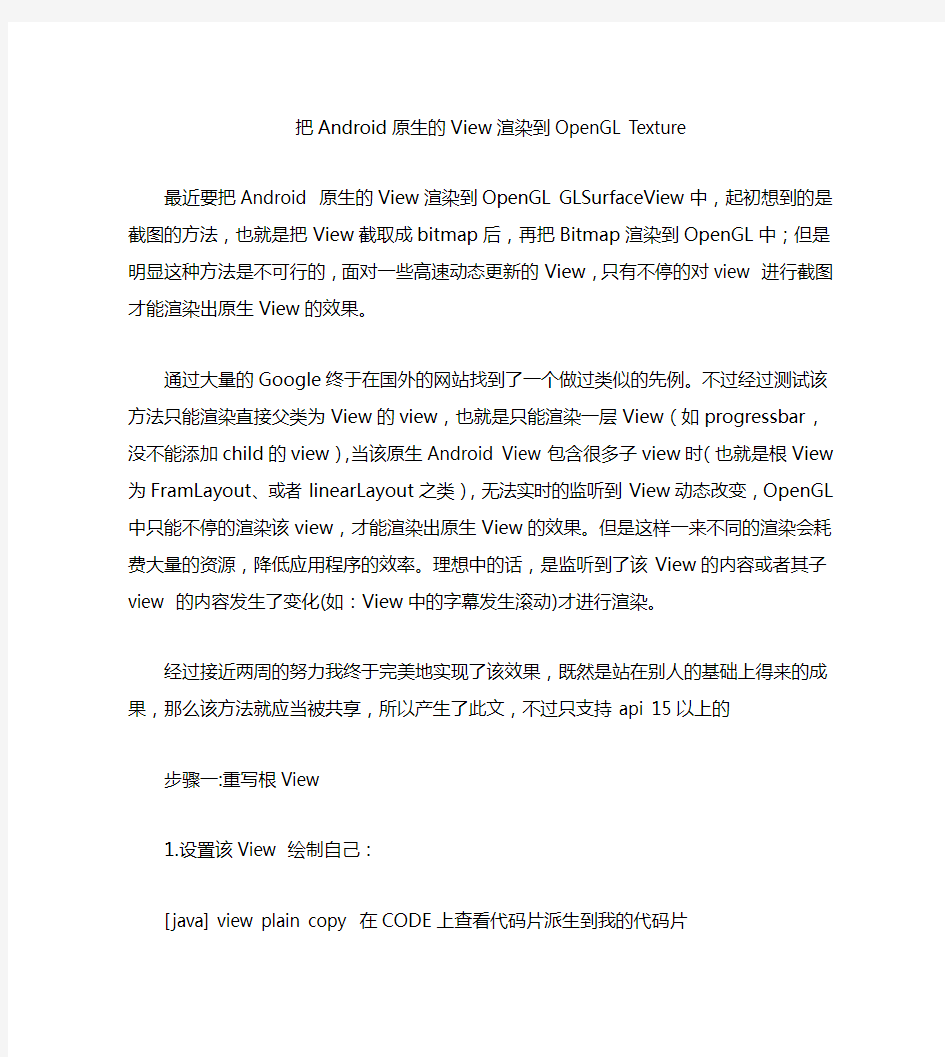

把Android原生的View渲染到OpenGL Texture
最近要把Android 原生的View渲染到OpenGL GLSurfaceView中,起初想到的是截图的方法,也就是把View截取成bitmap后,再把Bitmap 渲染到OpenGL中;但是明显这种方法是不可行的,面对一些高速动态更新的View,只有不停的对view 进行截图才能渲染出原生View的效果。
通过大量的Google终于在国外的网站找到了一个做过类似的先例。不过经过测试该方法只能渲染直接父类为View的view,也就是只能渲染一层View(如progressbar,没不能添加child的view),当该原生Android View包含很多子view时(也就是根View为FramLayout、或者linearLayout之类),无法实时的监听到View动态改变,OpenGL中只能不停的渲染该view,才能渲染出原生View的效果。但是这样一来不同的渲染会耗费大量的资源,降低应用程序的效率。理想中的话,是监听到了该View的内容或者其子view 的内容发生了变化(如:View中的字幕发生滚动)才进行渲染。
经过接近两周的努力我终于完美地实现了该效果,既然是站在别人的基础上得来的成果,那么该方法就应当被共享,所以产生了此文,不过只支持api 15以上的
步骤一:重写根View
1.设置该View 绘制自己:
[java] view plain copy 在CODE上查看代码片派生到我的代码片setWillNotDraw(false);
2.监听View的变化,重写View,用ViewTreeObServer来监听,方法如下:
[java] view plain copy 在CODE上查看代码片派生到我的代码片private void addOnPreDrawListener() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.HONEYCOMB) {
final ViewTreeObserver mObserver = getViewTreeObserver();
if (mObserver != null) {
mObserver.addOnPreDrawListener(new OnPreDrawListener() { @Override
public boolean onPreDraw() {
if (isDirty()) {//View或者子view发生变化
invalidate();
}
return true;
}
});
}
}
}
3.重写该View的onDraw方法:
[java] view plain copy 在CODE上查看代码片派生到我的代码片@Override
protected void onDraw(Canvas canvas) {
try {
if (mSurface != null) {
Canvas surfaceCanvas = mSurface.lockCanvas(null); super.dispatchDraw(surfaceCanvas);
mSurface.unlockCanvasAndPost(surfaceCanvas);
mSurface.release();
mSurface = null;
mSurface = new Surface(mSurfaceTexture);
}
} catch (OutOfResourcesException e) {
e.printStackTrace();
}
}
步骤二:GLSurfaceView.Renderer
[java] view plain copy 在CODE上查看代码片派生到我的代码片class CustomRenderer implements GLSurfaceView.Renderer { int glSurfaceTex;
private final int GL_TEXTURE_EXTERNAL_OES = 0x8D65;
long currentTime;
long previousTime;
boolean b = false;
int frameCount = 0;
DirectDrawer mDirectDrawer;
ActivityManager activityManager;
MemoryInfo _memoryInfo;
// Fixed values
private int TEXTURE_WIDTH = 360;
private int TEXTURE_HEIGHT = 360;
Context context;
private LauncherAppWidgetHostView addedWidgetView; private SurfaceTexture surfaceTexture = null;
private Surface surface;
float fps;
public CustomRenderer(Context context, LauncherAppWidgetHostView addedWidgetView, Display mDisplay){ this.context = context;
this.addedWidgetView = addedWidgetView;
TEXTURE_WIDTH = mDisplay.getWidth();
TEXTURE_HEIGHT = mDisplay.getHeight();
_memoryInfo = new MemoryInfo();
activityManager = (ActivityManager)
context.getApplicationContext().getSystemService(Context.ACTI VITY_SERVICE);
}
@Override
public void onDrawFrame(GL10 gl) {
synchronized (this) {
surfaceTexture.updateTexImage();
}
activityManager.getMemoryInfo(_memoryInfo);
GLES20.glClearColor(0.0f, 0.0f, 1.0f, 1.0f);
GLES20.glClear(GLES20.GL_DEPTH_BUFFER_BIT |
GLES20.GL_COLOR_BUFFER_BIT);
GLES20.glEnable(GLES20.GL_BLEND);
GLES20.glBlendFunc(GLES20.GL_ONE,
GLES20.GL_ONE_MINUS_SRC_ALPHA);
float[] mtx = new float[16];
surfaceTexture.getTransformMatrix(mtx);
mDirectDrawer.draw(mtx);
calculateFps();
//getAppMemorySize();
//getRunningAppProcessInfo();
//Log.v("onDrawFrame", "FPS: " + Math.round(fps) + ", availMem: " + Math.round(_memoryInfo.availMem / 1048576) + "MB");
}
private void getAppMemorySize(){
ActivityManager mActivityManager = (ActivityManager) context.getSystemService(Context.ACTIVITY_SERVICE);
android.os.Debug.MemoryInfo[] memoryInfos = mActivityManager.getProcessMemoryInfo(new
int[]{android.os.Process.myPid()});
int size = memoryInfos[0].dalvikPrivateDirty;
Log.w("getAppMemorySize", size / 1024 + " MB");
}
private void getRunningAppProcessInfo() {
ActivityManager mActivityManager = (ActivityManager) context.getSystemService(Context.ACTIVITY_SERVICE);
//获得系统里正在运行的所有进程
List
for (RunningAppProcessInfo runningAppProcessInfo : runningAppProcessesList) {
// 进程ID号
int pid = runningAppProcessInfo.pid;
// 用户ID
int uid = runningAppProcessInfo.uid;
// 进程名
String processName = runningAppProcessInfo.processName;
// 占用的内存
int[] pids = new int[] {pid};
Debug.MemoryInfo[] memoryInfo =
mActivityManager.getProcessMemoryInfo(pids);
int memorySize = memoryInfo[0].dalvikPrivateDirty;
System.out.println("processName="+processName+",currentPid: "+ "pid= "
+android.os.Process.myPid()+"----------->"+pid+",uid="+uid+", memorySize="+memorySize+"kb");
}
}
@Override
public void onSurfaceCreated(GL10 gl, EGLConfig config) { surface = null;
surfaceTexture = null;
glSurfaceTex = Engine_CreateSurfaceTexture(TEXTURE_WIDTH, TEXTURE_HEIGHT);
Log.d("GLES20Ext", "glSurfaceTex" + glSurfaceTex);
if (glSurfaceTex > 0) {
surfaceTexture = new SurfaceTexture(glSurfaceTex);
surfaceTexture.setDefaultBufferSize(TEXTURE_WIDTH, TEXTURE_HEIGHT);
surface = new Surface(surfaceTexture);
addedWidgetView.setSurface(surface);
addedWidgetView.setSurfaceTexture(surfaceTexture);
//addedWidgetView.setSurfaceTexture(surfaceTexture);
mDirectDrawer = new DirectDrawer(glSurfaceTex);
}
}
float calculateFps() {
frameCount++;
if (!b) {
b = true;
previousTime = System.currentTimeMillis();
}
long intervalTime = System.currentTimeMillis() - previousTime;
if (intervalTime >= 1000) {
b = false;
fps = frameCount / (intervalTime / 1000f);
frameCount = 0;
Log.w("calculateFps", "FPS: " + fps);
}
return fps;
}
int Engine_CreateSurfaceTexture(int width, int height) {
/*
* Create our texture. This has to be done each time the surface is
* created.
*/
int[] textures = new int[1];
GLES20.glGenTextures(1, textures, 0);
glSurfaceTex = textures[0];
if (glSurfaceTex > 0) {
GLES20.glBindTexture(GL_TEXTURE_EXTERNAL_OES, glSurfaceTex);
// Notice the use of GL_TEXTURE_2D for texture creation
GLES20.glTexImage2D(GLES20.GL_TEXTURE_2D, 0, GLES20.GL_RGB, width, height, 0, GLES20.GL_RGB, GLES20.GL_UNSIGNED_BYTE, null);
GLES20.glTexParameteri(GL_TEXTURE_EXTERNAL_OES,
GLES20.GL_TEXTURE_MIN_FILTER, GLES20.GL_NEAREST);
GLES20.glTexParameteri(GL_TEXTURE_EXTERNAL_OES,
GLES20.GL_TEXTURE_MAG_FILTER, GLES20.GL_NEAREST);
GLES20.glTexParameteri(GL_TEXTURE_EXTERNAL_OES,
GLES20.GL_TEXTURE_WRAP_S, GLES20.GL_CLAMP_TO_EDGE);
GLES20.glTexParameteri(GL_TEXTURE_EXTERNAL_OES,
GLES20.GL_TEXTURE_WRAP_T, GLES20.GL_CLAMP_TO_EDGE);
}
return glSurfaceTex;
}
@Override
public void onSurfaceChanged(GL10 gl, int width, int height) {
}
}
[java] view plain copy 在CODE上查看代码片派生到我的代码片public class DirectDrawer {
private final String vertexShaderCode =
"attribute vec4 vPosition;" +
"attribute vec2 inputTextureCoordinate;" +
"varying vec2 textureCoordinate;" +
"void main()" +
"{"+
"gl_Position = vPosition;"+
"textureCoordinate = inputTextureCoordinate;" +
"}";
private final String fragmentShaderCode =
"#extension GL_OES_EGL_image_external : require\n"+
"precision mediump float;" +
"varying vec2 textureCoordinate;\n" +
"uniform samplerExternalOES s_texture;\n" +
"void main() {" +
" gl_FragColor = texture2D( s_texture,
textureCoordinate );\n" +
"}";
private FloatBuffer vertexBuffer, textureVerticesBuffer;
private ShortBuffer drawListBuffer;
private final int mProgram;
private int mPositionHandle;
private int mTextureCoordHandle;
private short drawOrder[] = { 0, 1, 2, 0, 2, 3 }; // order to draw vertices
// number of coordinates per vertex in this array
private static final int COORDS_PER_VERTEX = 2;
private final int vertexStride = COORDS_PER_VERTEX * 4; // 4 bytes per vertex
static float squareCoords[] = {
-1.0f, 0.0f,
-1.0f, -2.2f,
1.0f, -
2.2f,
1.0f, 0.0f,
};
static float textureVertices[] = {
0f, 0f,
0f, 1f,
1f, 1f,
1f, 0f,
};
private int texture;
public DirectDrawer(int texture)
{
this.texture = texture;
// initialize vertex byte buffer for shape coordinates
ByteBuffer bb =
ByteBuffer.allocateDirect(squareCoords.length * 4);
bb.order(ByteOrder.nativeOrder());
vertexBuffer = bb.asFloatBuffer();
vertexBuffer.put(squareCoords);
vertexBuffer.position(0);
// initialize byte buffer for the draw list
ByteBuffer dlb = ByteBuffer.allocateDirect(drawOrder.length * 2);
dlb.order(ByteOrder.nativeOrder());
drawListBuffer = dlb.asShortBuffer();
drawListBuffer.put(drawOrder);
drawListBuffer.position(0);
ByteBuffer bb2 =
ByteBuffer.allocateDirect(textureVertices.length * 4);
bb2.order(ByteOrder.nativeOrder());
textureVerticesBuffer = bb2.asFloatBuffer();
textureVerticesBuffer.put(textureVertices);
textureVerticesBuffer.position(0);
int vertexShader = loadShader(GLES20.GL_VERTEX_SHADER, vertexShaderCode);
int fragmentShader = loadShader(GLES20.GL_FRAGMENT_SHADER, fragmentShaderCode);
mProgram = GLES20.glCreateProgram(); // create empty OpenGL ES Program
GLES20.glAttachShader(mProgram, vertexShader); // add the vertex shader to program
GLES20.glAttachShader(mProgram, fragmentShader); // add the fragment shader to program
GLES20.glLinkProgram(mProgram); //
creates OpenGL ES program executables
}
public void draw(float[] mtx)
{
GLES20.glUseProgram(mProgram);
GLES20.glActiveTexture(GLES20.GL_TEXTURE0);
GLES20.glBindTexture(GLES11Ext.GL_TEXTURE_EXTERNAL_OES, texture);
// get handle to vertex shader's vPosition member
mPositionHandle = GLES20.glGetAttribLocation(mProgram, "vPosition");
// Enable a handle to the triangle vertices
GLES20.glEnableVertexAttribArray(mPositionHandle);
// Prepare the
GLES20.glVertexAttribPointer(mPositionHandle,
COORDS_PER_VERTEX, GLES20.GL_FLOAT, false, vertexStride, vertexBuffer);
mTextureCoordHandle = GLES20.glGetAttribLocation(mProgram, "inputTextureCoordinate");
GLES20.glEnableVertexAttribArray(mTextureCoordHandle);
// textureVerticesBuffer.clear();
// textureVerticesBuffer.put( transformTextureCoordinates( // textureVertices, mtx ));
// textureVerticesBuffer.position(0);
GLES20.glVertexAttribPointer(mTextureCoordHandle, COORDS_PER_VERTEX, GLES20.GL_FLOAT, false, vertexStride, textureVerticesBuffer);
GLES20.glDrawElements(GLES20.GL_TRIANGLES,
drawOrder.length, GLES20.GL_UNSIGNED_SHORT, drawListBuffer);
// Disable vertex array
GLES20.glDisableVertexAttribArray(mPositionHandle);
GLES20.glDisableVertexAttribArray(mTextureCoordHandle);
}
private int loadShader(int type, String shaderCode){
// create a vertex shader type (GLES20.GL_VERTEX_SHADER)
// or a fragment shader type (GLES20.GL_FRAGMENT_SHADER)
int shader = GLES20.glCreateShader(type);
// add the source code to the shader and compile it
GLES20.glShaderSource(shader, shaderCode);
GLES20.glCompileShader(shader);
return shader;
}
private float[] transformTextureCoordinates( float[] coords, float[] matrix)
{
float[] result = new float[ coords.length ];
float[] vt = new float[4];
for ( int i = 0 ; i < coords.length ; i += 2 ) {
float[] v = { coords[i], coords[i+1], 0 , 1 };
Matrix.multiplyMV(vt, 0, matrix, 0, v, 0);
result[i] = vt[0];
result[i+1] = vt[1];
}
return result;
}
}
步骤三:配置GLSurfaceView:
[java] view plain copy 在CODE上查看代码片派生到我的代码片GLSurfaceView glSurfaceView = new
GLSurfaceView(getApplicationContext());
// Setup the surface view for drawing to
glSurfaceView.setEGLContextClientVersion(2);
glSurfaceView.setEGLConfigChooser(8, 8, 8, 8, 16, 0);
glSurfaceView.setRenderer(renderer);
//glSurfaceView.setZOrderOnTop(true);
// Add our WebView to the Android View hierarchy
glSurfaceView.setLayoutParams(new
https://www.360docs.net/doc/3e5392015.html,youtParams(https://www.360docs.net/doc/3e5392015.html,youtParams.MATCH_PAREN T, https://www.360docs.net/doc/3e5392015.html,youtParams.MATCH_PARENT));
android 自定义圆角头像以及使用declare-styleable进行配置属性解析
android 自定义圆角头像以及使用declare-styleable进行配置属性解析由于最新项目中正在检查UI是否与效果图匹配,结果关于联系人模块给的默认图片是四角稍带弧度的圆角,而我们截取的图片是正方形的,现在要给应用统一替换。应用中既用到大圆角头像(即整个头像是圆的)又用到四角稍带弧度的圆角头像,封装一下以便重用。以下直接见代码 [java] view plain copy 在CODE上查看代码片派生到我的代码片 package com.test.demo; import com.test.demo.R; import android.content.Context; import android.content.res.TypedArray; import android.graphics.Bitmap; import android.graphics.BitmapShader; import android.graphics.Canvas; import android.graphics.Matrix; import android.graphics.Paint; import android.graphics.RectF; import android.graphics.Shader.TileMode; import android.graphics.drawable.BitmapDrawable; import android.graphics.drawable.Drawable; import android.os.Bundle; import android.os.Parcelable; import android.util.AttributeSet; import android.util.Log; import android.util.TypedValue; import android.widget.ImageView; /** * 圆角imageview */ public class RoundImageView extends ImageView { private static final String TAG = "RoundImageView"; /** * 图片的类型,圆形or圆角 */ private int type; public static final int TYPE_CIRCLE = 0; public static final int TYPE_ROUND = 1; /** * 圆角大小的默认值
高通android平台开发
问题描述: 对于有过开发高通android系统的人来说,获取代码构建开发环境并不是难事,但对于刚刚接触这一块内容的人,如果没有详细的说明很容易走弯路,本文档就是根据本人的实践总结的一些经验教训。 1.代码获取 高通的android代码分为两部分,一部分是开源的,可以从网站https://https://www.360docs.net/doc/3e5392015.html,/xwiki/bin/QAEP/下载,需要知道要下载的代码的分支及build id。另一部分是非开源的,需要从高通的另一个网站https://https://www.360docs.net/doc/3e5392015.html,/login/上下载,这个下载是有权限限制的,晓光的帐号可以下载代码。后面这部分代码需要放到第一部分代码的vendor指定目录下,可能是vendor/qcom-proprietary或vendor/qcom/proprietary,根据版本的不同有所区别。 高通平台相关的东西基本都在vendor/qcom/proprietary下或device/qcom下 2.编译环境构建(ubuntu 10.04 64位) Android2.3.x后的版本需要在64位下进行编译 更新ubuntu源,要加上deb https://www.360docs.net/doc/3e5392015.html,/ lucid partner 这个 源用来安装java。 apt-get install git-core gnupg flex bison gperf build-essential zip curl zlib1g-dev x11proto-core-dev libx11-dev libxml-simple-perl sun-java6-jdk gcc-multilib g++-multilib libc6-dev-i386 lib32ncurses5-dev ia32-libs lib32z-dev lib32readline5-dev 研发主机不能更新java,需要让IT安装sun-java6-jdk。 在命令行执行sudo dpkg-reconfigure dash 选择no,否则编译时会报一下脚本语法错误 编译的过程中https://https://www.360docs.net/doc/3e5392015.html,/xwiki/bin/QAEP/和版本的 release notes中都有介绍,首先source build/envsetup.sh,然后choosecombo选择需要的选项,最后make或make –j4。-j4用来指定参与编译的cpu个数,指定了编译会快些。编译单个模块的时候只需要在make后面跟 上模块的名字 为了简化可以使用以下脚本 export TARGET_SIMULATOR=fasle export TARGET_BUILD_TYPE=release export TARGET_PRODUCT=msm7627a export TARGET_BUILD_VARIANT=eng set_stuff_for_environment make $1 编译的中间结果在out/target/product/平台/obj目录下,有时候为了完全
A20_Android开发手册_V1[1].0
C o n f i d e n t i a l A20Android 开发手册 V 1.0 2013-02013-03 3-15
C o n f i d e n t i a l Revision History Version Date Section/Page Changes 1.0 2013-03-15 初始版本
C o n f i d e n t i a l 目录 一、A20概述 (4) 1.1A20主控介绍.....................................................................................................................51.2外围设备介绍.....................................................................................................................51.3软件资源介绍.....................................................................................................................5二、建立开发环境. (5) 2.1硬件资源............................................................................................................................62.2软件资源. (6) 2.2.1安装JDK (ubuntu12.04).....................................................................................62.2.2安装平台支持软件(ubuntu12.04).....................................................................62.2.3安装编译工具链(ubuntu12.04).........................................................................62.2.4安装phoenixSuit (windows xp )........................................................................72.2.5其他软件(windows xp ).. (7) 三、源码下载 (8) 3.1wing 源码下载....................................................................................................................83.2仓库的目录树.. (8) 3.2.1android 目录树.........................................................................................................83.2.2lichee 目录结构.. (9) 3.2.2.1buildroot 目录结构........................................................................................93.2.2.2linux-3.3目录结构......................................................................................103.2.2.3u-boot 目录结构..........................................................................................113.2.2.4tools 目录结构............................................................................................123.2.2.5boot 目录结构 (12) 四、编译和打包 (13) 4.1源码编译 (13) 4.1.1lichee 源码编译......................................................................................................134.1.2android 源码编译...................................................................................................134.2打包固件.. (13) 4.2.1完全打包...............................................................................................................134.2.2局部打包 (14) 五、固件烧写 (14) 5.1使用PhoenixSuit 烧写固件.............................................................................................145.2使用fastboot 更新系统 (14) 5.2.1进入fastboot 模式.................................................................................................145.2.2fastboot 命令使用.. (15) 六、recovery 功能使用 (15) 6.1键值的查看......................................................................................................................156.2按键选择..........................................................................................................................166.3功能使用..........................................................................................................................16七、调试 (17) 7.1调试apk...........................................................................................................................177.2调试linux 内核. (17)
Android平台我的日记设计文档
Android平台我的日记 设计文档 项目名称:mydiray 项目结构示意: 阶段任务名称(一)布局的设计 开始时间: 结束时间: 设计者: 梁凌旭 一、本次任务完成的功能 1、各控件的显示 二、最终功能及效果 三、涉及知识点介绍 四、代码设计 activity_main.xml: android:layout_centerHorizontal="true" android:layout_marginTop="88dp" android:text="@string/wo" android:textSize="35sp"/> Android开发参考文档 一、Android编码规范 1. java代码中不出现中文,最多注释中可以出现中文.xml代码中注释 2. 成员变量,局部变量、静态成员变量命名、常量(宏)命名 1). 成员变量: activity中的成员变量以m开头,后面的单词首字母大写(如Button mBackButton; String mName);实体类和自定义View的成员变量可以不以m开头(如ImageView imageView,String name), 2). 局部变量命名:只能包含字母,组合变量单词首字母出第一个外,都为大写,其他字母都为小写 3). 常量(宏)命名: 只能包含字母和_,字母全部大写,单词之间用_隔开UMENG_APP_KEY 3. Application命名 项目名称+App,如SlimApp,里面可以存放全局变量,但是杜绝存放过大的实体对象4. activity和其中的view变量命名 activity命名模式为:逻辑名称+Activity view命名模式为:逻辑名称+View 建议:如果layout文件很复杂,建议将layout分成多个模块,每个模块定义一个moduleViewHolder,其成员变量包含所属view 5. layout及其id命名规则 layout命名模式:activity_逻辑名称,或者把对应的activity的名字用“_”把单词分开。 命名模式为:view缩写_模块名称_view的逻辑名称, 用单词首字母进行缩写 view的缩写详情如下 LayoutView:lv RelativeView:rv TextView:tv ImageView:iv ImageButton:ib Button:btn 6. strings.xml中的 1). id命名模式: activity名称_功能模块名称_逻辑名称/activity名称_逻辑名称/common_逻辑名称,strings.xml中,使用activity名称注释,将文件内容区分开来 2). strings.xml中使用%1$s实现字符串的通配,合起来写 7. drawable中的图片命名 命名模式:activity名称_逻辑名称/common_逻辑名称/ic_逻辑名称 (逻辑名称: 这是一个什么样的图片,展示功能是什么) 8. styles.xml 将layout中不断重现的style提炼出通用的style通用组件,放到styles.xml中; 9. 使用layer-list和selector,主要是View onCclick onTouch等事件界面反映 android 自定义控件的过程 invalidate()会导致computeScroll()以及onDraw()方法的执行computeScroll()方法是在屏幕流动的时候不停的去调用,scrollTo(int x,int y)则是滚动到相应的位置; scrollBy(int x, int y)则是移动一些距离,X为正是向左移动,为负时向右移动,Y与X的意义一个,只是是上下移动而已View对象显示在屏幕上,有几个重要步骤: 1.构造方法创建对象 2.测量View的大小onMeasure(int,int); 3.确定View的位置,View自身有一些权,决定权在父View手中. onLayout();基本上不常用,在继承View的时候基本上用不着,但在继承ViewGroup的时候的就要用到了,因为要对View进行布局,确定View的位置,确定的时候使用 指定子View的位置,左,上,右,下,是指在ViewGroup坐标系中的位置https://www.360docs.net/doc/3e5392015.html,yout(int xtop,int ytop, int xbottom, int ybottom); 4.绘制View的内容onDraw(Canvas) 实现过程: 1、构造方法: /** * 在布局文件中声名的view,创建的时候由系统调用 * * @param context * 上下文对象 * @param attrs * 属性集 */ public MyToggleButton(Context context, AttributeSet attrs) { super(context, attrs); initView(); } 2、测量View的大小: /** * 测量尺寸时的回调方法 */ Android开发环境搭建 在网上找各种解决办法,无奈,都是互相转帖,要错大家一起错,而且都是老版本的安装方法,虽然没有太大差别,但小的差异还是搞得人很头痛,因为有时候就是一点不同就要找好长时间解决方法,我现在把我的安装历程发布出来,供大家分享,当然版本再更新的话我也不能保证就完全正确,只能保证现在的版本完全没问题。因为现在的android版本与原来的版本有一定的区别,所以我在2.2的基础上改了一下,现在可以安装到4.0 安装JDK 这个在上一篇中已经介绍过了,注意JDK的版本为1.5或者1.6都可以就行了,推荐使用1.6版本,因为它要比以前快得多。还有JAVA_HOME的设置。用set JAVA_HOME命令检查一下就行了。 SDK 4.0安装 今年Google离开大陆,导致登陆他们的网站会比较麻烦,一般情况下是登陆不上去的,需要翻墙,大家都懂的,我不多说,如果能登上 https://www.360docs.net/doc/3e5392015.html,/sdk/index.html那是最好,如果登不上也没关系,能下的地方好多,上百度上搜有很多,而且下载都很快,我下载的是 android-sdk_r07-windows.zip,一下安装也是以它为例。 下载好后,随便解压到一个地方,目录结构如图所示: 此处SDK Manager.exe和以前版本略有区别,以前是SDK Setup.exe,功能都一样,双击它。(我们下载的也不是完整的SDK,只是一个安装SDK的工具) 双击之后我们会看到如下的界面: 左侧是我们要安装的SDK目录,如果你的网络够快而且空间不是特别少的话,选择Accept All,然后Install,开始漫长的在线安装: Android进阶——自定义View之自己绘 制彩虹圆环调色板 引言 前面几篇文章都是关于通过继承系统View和组合现有View来实现自定义View的,刚好由于项目需要实现一个滑动切换LED彩灯颜色的功能,所以需要一个类似调色板的功能,随着手在调色板有效区域滑动,LED彩灯随即显示相应的颜色,也可以通过左右的按钮,按顺序切换显示一组颜色,同时都随着亮度的改变LED彩灯的亮度随即变化,这篇基本上把继承View重绘实现自定义控件的大部分知识总结了下(当然还有蛮多没有涉及到,比如说自适应布局等),源码在Github上 一、继承View绘制自定义控件的通用步骤 自定义属性和继承View重写onDraw方法 实现构造方法,其中public RainbowPalette(Context context, AttributeSet attrs) 必须实现,否则无法通过xml引用,public RainbowPalette(Context context) ,public RainbowPalette(Context context, AttributeSet attrs, int defStyleAttr)可选,通常在构造方法中完成属性和其他成员变量的初始化 重写onMeasure方法,否则在xml中有些设置布局参数无效 @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { super.onMeasure(width, height);//重新设置View的位置,若不重写的话,则不会布局,即使设置centerInParent为true也无效 //setMeasuredDimension(width,height); } 手动调用invalidate或者postInvalidateon方法完成界面刷新 重写onTouchEvent方法实现基本的交互 定义回调接口供外部调用 二、彩虹圆环调色板设计思想 中国地质大学 毕业设计(论文)开题报告 题目:基于Android平台的浏览器开发与实现 学院:机电学院 专业:通信工程 班级:075083班 学号:858 姓名:许磊 指导教师:张晓峰 日期:2012 年 2 月29 日 一、选题的依据及意义: 随着智能手机的快速普及,智能手机操作系统市场风生水起。为了让智能手机用户能够随时随地查询互联网所提供的服务,一种高效的办法就是将应用系统的功能拓展到手机终端上,让手机能够通过移动网以及互联网访问Web网站并处理各种各样的业务。 浏览器是网民进入互联网的第一窗口,让用户能够快速的访问自己所需要的信息,如小说、新闻、音乐、视频、体育赛事、天气情况、股市行情等。如今,手机浏览器不仅仅是一种网络应用程序,也逐渐成为一种网络应用平台。各种网络应用基本都可以在浏览器上实现,所以被用户广泛接受成为各大浏览器厂商的最主要目标。 研究本课题的意义是让用户有一个快速的上网浏览入口,给用户一个界面友好,功能强大,易于操作,便于管理的浏览器。同时也让自己更加熟悉Android 应用程序的开发,了解Android应用程序开发的流程,各个模块之间的联系。 二、国内外研究现状及发展趋势: Android 是Google开发的基于Linux平台的开源手机操作系统。它包括操作系统、用户界面和应用程序—移动电话工作所需的全部软件,而且不存在任何以往阻碍移动产业创新的专有权障碍。Google与开放手机联盟合作开发了Android,这个联盟由包括中国移动、摩托罗拉、高通、宏达电和 T-Mobile 在内的30多家技术和无线应用的领军企业组成。Google通过与运营商、设备制造商、开发商和其他有关各方结成深层次的合作伙伴关系,希望借助建立标准化、开放式的移动电话软件平台,在移动产业内形成一个开放式的生态系统。 经过多年的发展,第三代数字通信( 3G)技术获得了广泛的接受,它为移动终端用户带来了更快的数据传输速率。随着3G网络的使用,移动终端不再仅是通讯网络的终端,还将成为互联网的终端。因此,移动终端的应用软件和需要的服务将会有很大的发展空间。Android用户也逐渐增加,根据发展趋势,Android将成为第一大智能手机平台。 浏览器作为用户访问网络的窗口,各大厂商也相继推出了自己的浏览器,如 安卓开发环境搭建完全手册 小安:博士,刚才听你说了这么多,我感觉Android平台真是前景无限,决定要从事Android开发,成为一名Android高手。今后请您多多指教! 大致:小安,我代表全体Android开发人员欢迎你加入Android开发的队伍,现在我就告诉你开发Android需要准备些什么。 1.1准备所需软件 1.1.1Android开发需要的工具 1)JDK5或JDK6 需要注意的是仅有JRE是不够的。JRE是Java的运行环境,而JDK不仅包含了JRE,还包含了我们开发Java程序所需要的工具集合。 JDK可以到:https://www.360docs.net/doc/3e5392015.html,/javase/downloads/index.jsp页面下载。 2)Eclipse3.5 使用MyEclipse也可以,但由于MyEclipse是收费的并且插件较多影响运行速度,因此不建议采用。Eclipse是一个开放源代码的、基于Java的可扩展的集成开发环境(IDE)。Eclipse中可以集成进多种插件,以完成特定语言的开发。 下载地址:https://www.360docs.net/doc/3e5392015.html,/downloads/页面中的EclipseIDEforJavaEEDevelopers链接。 3)Android SDK: SDK是我们开发Android应用程序的软件开发工具包。 下载地址:https://www.360docs.net/doc/3e5392015.html,/android/android-sdk_r05-windows.zip 4)Eclipse的插件ADT(Android Development Tools) Android开发工具(ADT)是一个为EclipseIDE设计的旨在提供一个强大的、集成的环境来建立Android 应用程序的插件。ADT扩展了Eclipse的功能,可以快速建立新的Android项目,创建一个应用程序界面。它添加了基于Android框架API的组件,使用AndroidSDK工具调试你的应用程序,甚至导出签名(或未签名)APKs以分发你的应用程序。在Eclipse中强烈建议使用ADT进行开发,ADT提供了令人难以置信的提高开发Android应用程序的效率。 下载地址:https://www.360docs.net/doc/3e5392015.html,/android/ADT-0.9.5.zip 准备好这些工具,我们就可以安装这些软件来搭建Android的开发环境了。有一点需要注意,以上的链接部分会由于官方的更新而产生变动,有时下载到的版本不同,但下载的方式如此,如果遇到问题可以参考官方帮助文档 1.2安装所需的软件 1.2.1.安装JDK6 1.找到JDK安装文件,双击运行,界面如下: 自定义Dialog; dialog = new Dialog(this); dialog.setContentView(https://www.360docs.net/doc/3e5392015.html,yout.by_baseinfo); dialog.setTitle("dialog的title"); /* * 获取Dialog的窗口对象及参数对象以修改对话框的布局设置, 可以直接调用this.getWindow(),表示获得这个Activity的Window * 对象,这样这可以以同样的方式改变这个Activity的属性. * Activity不可见时getWindow()返回值为null; */ Window dialogWindow = dialog.getWindow(); // 对话框的布局设置参数; https://www.360docs.net/doc/3e5392015.html,youtParams layoutParams = dialogWindow.getAttributes(); // 设置Window中的内容为左上对齐; dialogWindow.setGravity(Gravity.LEFT | Gravity.TOP); /* * lp.x与lp.y表示相对于原始位置的偏移. * 当参数值包含Gravity.LEFT时,对话框出现在左边,所以lp.x就表示相对左边的偏移,负值忽略. * 当参数值包含Gravity.RIGHT时,对话框出现在右边,所以lp.x就表示相对右边的偏移,负值忽略. * 当参数值包含Gravity.TOP时,对话框出现在上边,所以lp.y就表示相对上边的偏移,负值忽略. * 当参数值包含Gravity.BOTTOM时,对话框出现在下边,所以lp.y就表示相对下边的偏移,负值忽略. * 当参数值包含Gravity.CENTER_HORIZONTAL时 * ,对话框水平居中,所以lp.x就表示在水平居中的位置移动lp.x像素,正值向右移动,负值向左移动. * 当参数值包含Gravity.CENTER_VERTICAL时 * ,对话框垂直居中,所以lp.y就表示在垂直居中的位置移动lp.y像素,正值向右移动,负值向左移动. * gravity的默认值为Gravity.CENTER,即Gravity.CENTER_HORIZONTAL | * Gravity.CENTER_VERTICAL. * * 本来setGravity的参数值为Gravity.LEFT | Gravity.TOP时对话框应出现在程序的左上角,但在 * 我手机上测试时发现距左边与上边都有一小段距离,而且垂直坐标把程序标题栏也计算在内了, Gravity.LEFT, Gravity.TOP, * Gravity.BOTTOM与Gravity.RIGHT都是如此,据边界有一小段距离 */ // 相对于屏幕原位置(加上标题栏) 的偏移量; lp.x = 100; // 新位置X坐标 通信实训报告 -Android移动平台开发 学院:信息工程学院 班级: 学号: 姓名: 实训内容: 一.1.Andriod的简介 Android。2011年初数据显示,仅正式上市两年的操作系统Android已经超越称霸十年的塞班系统,使之跃居全球最受欢迎的智能手机平台。现在,Android系统不但应用于智能手机,也在平板电脑市场急速扩张,在智能MP4方面也有较大发展。采用Android系统主要厂商包括台湾的HTC,(第一台谷歌的手机G1由HTC生产代工)美国摩托罗拉,SE等,中国大陆厂商如:魅族(M9),华为、中兴、联想、蓝魔等。 二.1软件下载 Android SDK,网址是 . JDK的下载地址。 Eclipse的下载网址是 第一步:下载Android SDK 网址是 选择"Available Packages",选择想安装的版本(我是全选了,省事),然后单击“Install Selected”->选择“Accept All”,再单击“Install Accepted”就可以在线安装了。 第二步:安装JDK A下载JDK。Android SDK需要JDK5 以上的版本。JDK6的下载地址。 B安装JDK。下载完成后进行正常双击、安装,这里就不说了。。。 C配置Java环境变量 1配置Java Home。复制Java的安装路径,右键单击“我的电脑”->“属性”->“高级”—>“环境变量”中新建环境变量java_home,变量值为java安装路径。 2配置Path。右键单击“我的电脑”->“属性”->“高级”—>“环境变量”,在“系统环境变量”中编辑Path便来了个,添加Java的bin目录到其中。变量与变量中间使用分号“;”分隔。 3配置classpath。右键单击“我的电脑”->“属性”->“高级”—>“环境变量”,在“系统环境变量”中新建一个系统变量名称为“classpath”,变量值为半角句号“.” 第三步:下载Eclipse RK28平台技术文档 Android 开发入门 部 门: 内核组 版 本: V1.1 作 者: 陈美友 文件状态: [√] 草稿 [ ] 正式发布 [ ] 正在修改 日 期: 2008-12-1 关键字 Linux 、Android 、Native C/C++、编译、下载 概 述 本文档主要讲述了Android 的下载、编译,以 及本地C/C++程序的开发、Android 应用程序 的开发等… 本文档适用了Android 开发的初学者 目录 下载ANDROID源码 (3) 编译ANDROID源码: (4) 编译ANDROID中的LINUX内核: (5) 编译NATIVE C/C++程序 (6) 运行NATIVE C/C++应用程序 (7) 在WINDOWS XP操作系统上构建ANDROID应用程序开发环境 (8) 编写ANDROID应用程序 (9) ANDROID SDK文件夹结构分析 (9) ANDROID源代码结构 (10) ANDROID应用程序生成 (10) 注: 如果你要创建Android的SDK,那么你必须安装JDK5,不要安装JDK6 $sudo apt-get install sun-java5-jdk 如果你使用apt-get下载安装程序时,发现某些安装包无法下载的情况, 那么你可以从网络上其它地方用其它的工具下载安装包,然后进行安装。我 通常是通过Windows上的迅雷下载,然后通过VMware中的文件共享传到Linux系统中。 我的电脑: 主系统:Windows XP 模拟器安装在Windows XP上 VMware中安装Linux:Ubuntu8.10 Android源码存放路径:~/mydroid 下载Android源码 在这里面以Ubuntu(x86)操作系统为例,说明下载Android的步骤: 下载必要工具: 下载GIT工具: $ sudo apt-get install git-core gnupg 下载JDK6: $ sudo apt-get install sun-java6-jdk 下载下列工具包:flex, bison, gperf, libsdl-dev, libesd0-dev, libwxgtk2.6-dev (optional), build-essential, zip, curl $ sudo apt-get install flex bison gperf libsdl-dev libesd0-dev libwxgtk2.6-dev build-essential zip curl libncurses5-dev zlib1g-dev 下载Valgrind工具(可选): $ sudo apt-get install valgrind 安装Repo工具: 创建目录存放Repo: $ cd ~ $ mkdir bin $ export PATH=~/bin:$PATH 下载Repo: $ curl https://www.360docs.net/doc/3e5392015.html,/repo >~/bin/repo 今天和大家分享下组合控件的使用。很多时候android自定义控件并不能满足需求,如何做呢?很多方法,可以自己绘制一个,可以通过继承基础控件来重写某些环节,当然也可以将控件组合成一个新控件,这也是最方便的一个方法。今天就来介绍下如何使用组合控件,将通过两个实例来介绍。 第一个实现一个带图片和文字的按钮,如图所示: 整个过程可以分四步走。第一步,定义一个layout,实现按钮内部的布局。代码如下: 1. 2. 1了解Android系统 1.1 Android系统介绍 Android是Google 开发的基于Linux 平台的、开源的、智能手机操作系统。Android 包括操作系统、中间件和应用程序,由于源代码开放,Android 可被移植到不同的硬件平台上。 围绕在Google的Android 系统中,形成了移植开发和应用程序开发两个不同的开发方面。手机厂商从事移植开发工作,应用程序开发可以由任何单位和个人完成,开发的过程可以基于真实的硬件系统,还可以基于仿真器环境。 作为一个手机平台,Android 在技术上的优势主要有以下几点: ●全开放智能手机平台 ●多硬件平台的支持:应用程序可通过标准API访问核心移动设备功能。 ●使用众多的标准化技术:可以轻松的嵌入HTML、JavaScript等网络内容 ●核心技术完整,统一:应用程序是平等条件创建的,可被替换或扩展。 ●应用程序可以并行运行。Android是完整的多任务环境,在后台运行时,应用程 序可生成通知引起用户注意。 ●完善的SDK 和文档 ●完善的辅助开发工具 Android 的开发者可以在完备的开发环境中进行开发,Android 的官方网站也提供了丰富的文档、资料。这些都使得Android 系统的开发和运行在一个良好的生态环境中。 1.2 Android系统的软件结构 Android 是一个开放的软件系统,它包含了众多的源代码。从下至上,Android 系统分成4个层次: ●第1 层次:Linux 操作系统及驱动; ●第2 层次:本地代码框架,包含各种类库和运行环境; ●第3 层次:Java 框架; ●第4 层次:Java 应用程序。 Android 的第1 层次由C 语言实现,第2层次由C 和/C++实现,第3、4层次主要由Java代码实现。对于Android 应用程序的开发,主要关注第3层次和第4层次之间的接口。 Android 系统的架构如图所示: android自定义View之Android手机通讯录制作 我们的手机通讯录一般都有这样的效果,如下图: OK,这种效果大家都见得多了,基本上所有的Android手机通讯录都有这样的效果。那我们今天就来看看这个效果该怎么实现。 一.概述 1.页面功能分析 整体上来说,左边是一个ListView,右边是一个自定义View,但是左边的ListView 和我们平常使用的ListView还有一点点不同,就是在ListView中我对所有的联系人进行了分组,那么这种效果的实现最常见的就是两种思路: 1.使用ExpandableListView来实现这种分组效果 2.使用普通ListView,在构造Adapter时实现SectionIndexer接口,然后在Adapter 中做相应的处理 这两种方式都不难,都属于普通控件的使用,那么这里我们使用第二种方式来实现,第一种方式的实现方法大家可以自行研究,如果你还不熟悉ExpandableListView的使用,可以参考我的另外两篇博客: 1.使用ExpandableListView实现一个时光轴 2.android开发之ExpandableListView的使用,实现类似QQ好友列表 OK,这是我们左边ListView的实现思路,右边这个东东就是我们今天的主角,这里我通过自定义一个View来实现,View中的A、B......#这些字符我都通过canvas的drawText 方法绘制上去。然后重写onTouchEvent方法来实现事件监听。 2.要实现的效果 要实现的效果如上图所示,但是大家看图片有些地方可能还不太清楚,所以这里我再强调一下: 1.左边的ListView对数据进行分组显示 2.当左边ListView滑动的时候,右边滑动控件中的文字颜色能够跟随左边ListView 的滑动自动变化 3.当手指在右边的滑动控件上滑动时,手指滑动到的地方的文字颜色应当发生变化,同时在整个页面的正中央有一个TextView显示手指目前按下的文字Android开发规范参考文档
android 自定义控件的过程
Android4.0开发环境搭建
Android进阶——自定义View之自己绘制彩虹圆环调色板
基于Android平台的浏览器开发与实现
安卓开发环境搭建完全手册
android自定义布局或View
Android简单的登陆界面的设计开发
Android开发入门文档 v1.1
Android自定义控件
1 Android系统简介及开发环境的搭建
android自定义View之Android手机通讯录制作