计算机科学与技术外文翻译
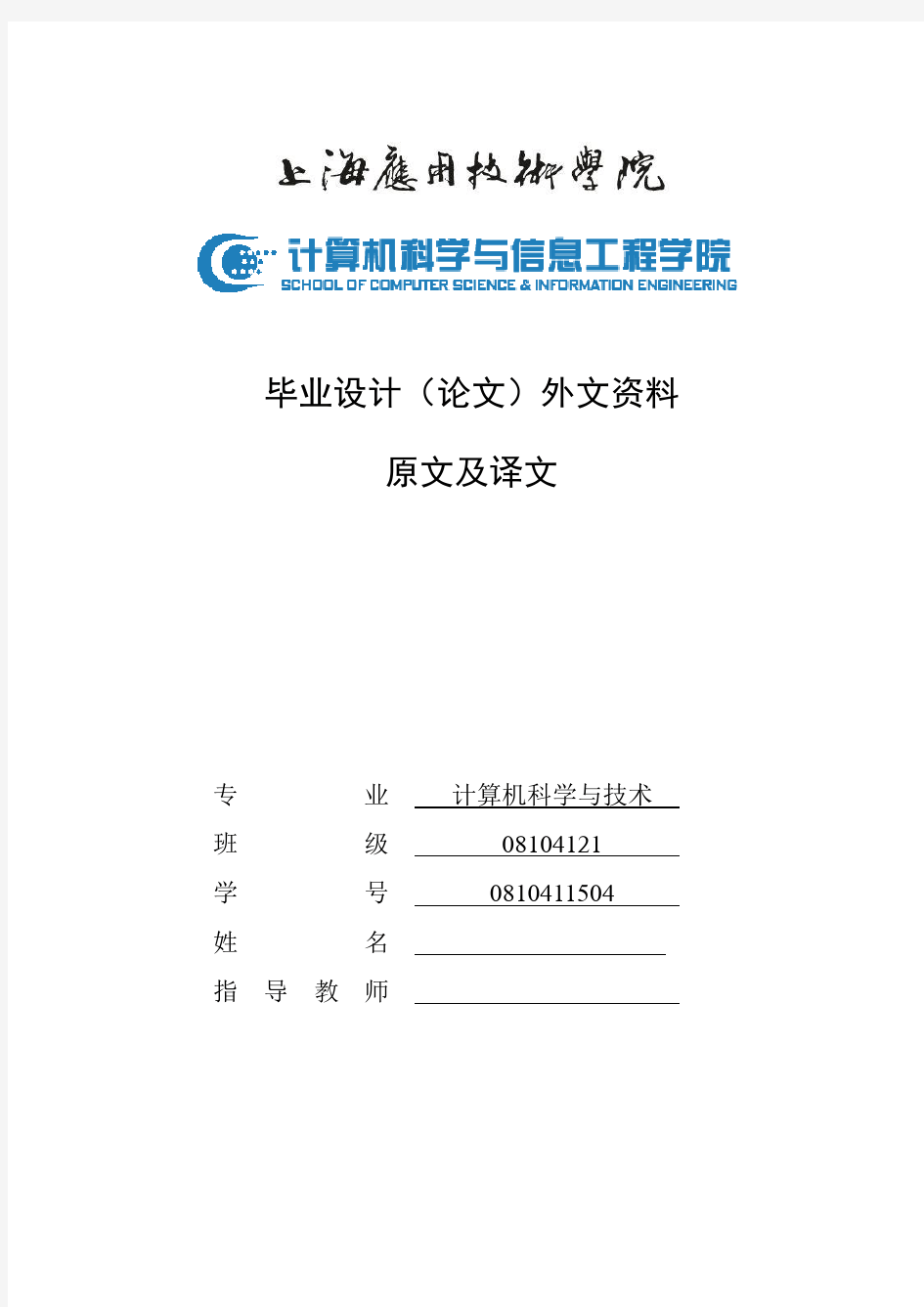

毕业设计(论文)外文资料
原文及译文
专业计算机科学与技术班级08104121
学号0810411504
姓名
指导教师
原文出处:Flash 8 ActionScript Bible, Joey Lott and Robert Reinhardt, Published by Wiley Publishing, Inc.
Flash 8 ActionScript Bible
Joey Lott and Robert Reinhardt
Understanding Datatypes
When we talk about data, we‘re talking about information or values. These values can be of many types. For example, even in a very simple movie you might still have a number, some text, and a MovieClip instance. All three of these examples are data of different types —what ActionScript calls datatypes.
Flash is actually capable of performing datatype conversions when necessary. However, this can lead to some poor coding practices on the part of ActionScript developers. For this reason, the ActionScript 2.0 standards require that you pay closer attention to the datatypes you are using.
In ActionScript, you‘ll work with many different datatypes. However, for the sak e of understanding how these datatypes work, you can consider them in two basic categories: primitive types and reference types. The primitive types are called primitive because they are the basic foundational datatypes, not because they lack importance. The reference datatypes are called reference types because they reference the primitive types.
Primitive datatypes include strings, numbers, Booleans, undefined, and null. We‘ll examine each of these primitive datatypes a little more closely in this chapter. Reference datatypes are all objects, which is the subject of much of the rest of this book, so we‘ll defer the majority of the discussion of reference datatypes to those later chapters.
Working with Strings
Strings are characters or words. String values must always be enclosed in either single quotes or double quotes. Here are a few examples of strings:
“a”
‘b’
“1”
“Joey”
‘123’
‘abc’
“****”
Strings are used whenever you want to work with characters or words. For example, you can use strings to populate text fields in your movie, or you can use strings to programmatically create names for new MovieClip instances. You‘ve also already seen how
strings can be used with actions such as trace(). The trace() action requires that you provide it with a message to display in the Output panel. That message must evaluate to a string value.
trace(“I know him; Marley’s Ghost!”);
As already mentioned, you can use either double quotes or single quotes when defining a string value. Which you choose is often purely a matter of personal preference. There are two rules that you must follow, however, if you want your code to work without error. First, you must have a matching closing quote for every opening quote. And whichever type of quote you use to open the string literal must be used to close it. In other words, mismatched quotes are not allowed. Here are two examples of correctly matched quotes on string literals: “here is a string”
‘here is a string’
And here are three examples of incorrect quotes on string literals:
“here is a string’
‘here is a string”
“here is a string
There are times when more than personal preference might dictate which type of quotes you choose to use. Notice what would happen if you tried the following:
trace(‘I know him; Marley’s Ghost!’);
This line of code would actually cause an error because it would interpret the apostrophe as the closing quote and then fail to know what to do with the remainder of the string. This is easily remedied by using double quotes around the string:
trace(“I know him; Marley’s Ghost!”);
The inverse is true as well, of course — if you want to use a double quotation mark as a character in a string literal, you can use single quotes around the entire value. The problem arises when you want to use both single and double quotation marks as characters within a string literal. There is an easy way to accommodate this: by using special characters. To learn more about special characters, see Chapter 11.
Working with Numbers
In Flash, all numbers are treated as the number datatype. Positive, negative, floating point, integer, and so forth, are all simply considered numbers, with no further differentiation generally required on your part.
To define a number, you need only type the number without any quotes. The following are examples of numbers.
6
12
-1
3.3
1.8
Number datatypes allow you to perform all kinds of mathematical operations, as shown in the following examples:
trace(5 + 5); // Displays: 10
trace(5 –4); // Displays: 1
trace(5 / 2); // Displays: 2.5
It is important to understand when to use numbers and when to use strings. If you try to use a string when you really want to use a number, you can end up with unexpected results. For example:
trace(“5”+ “5”); // Displays: 55
In the example, the resulting value of addedAges is “55”, not 10 (as you may expect). This is because ActionScript treats the two values as strings and concatenates them, rather than adding the numeric values.
However, it should also be understood that there are legitimate reasons for numbers to be treated as strings. For example, even though a phone number is composed of numbers, it is usually treated as a string. And if you want to format the phone number with spaces, parentheses, dashes, and so on, it is necessary that the phone number be treated as a string by enclosing the value in quotation marks.
Using Booleans
Boolean data is data that can hold only two values: true and false. Boolean variables are used to test conditions within your Flash movie. Boolean values are used commonly in conditional expressions within statements such as the if statement and control structures such as the for and while statements. For more information about conditional statements and control structures, read the section ―Using Statements That Control Flow: Control Structures‖ la ter in this chapter.
Understanding the undefined and null Datatypes
ActionScript has two additional primitive datatypes — undefined and null — with which you‘ll want to familiarize yourself. In order to have a better understanding of these datatypes, it is useful to first be familiar with variables. Therefore, we‘ll interweave the discussion of these two datatypes with the discussion of variables later in this chapter.
Casting Data
ActionScript allows you to tell Flash to convert a value to a specific datatype by what is known as casting. When you cast a value, you use the following syntax:
Datatype(value)
For example, you can cast a string to a number as follows:
Number(“123”)
When you are casting data you have to be careful. Some casting will have unexpected results. For example, the numeric value of 0 can be converted to a Boolean false, and any nonzero value will be converted to true. For example:
trace(Boolean(0)); // Displays: false
trace(Boolean(1)); // Displays: true
Therefore, if you convert the string values of true and false, they will both convert to the Boolean true value because both string values are nonzero values.
trace(Boolean(“true”)); // Displays: true
trace(Boolean(“false”)); // Displays: true
Using Variables
In Flash movies you‘re going t o be using a lot of data. Now, with all that data floating around, you‘re going to want some way to keep track of it. This is where variables become very useful.
A variable is a named container that you can use to hold or reference some particular data. Once you have created a variable, you can store and retrieve data in the variable. Although a variable can contain only one value at a time, you can use the same variable to contain or reference different data at different times. For example, if you create a variable named nYear, it may contain the value of 2005 at one point, but at another point it may contain the value 2006.
Consider a rental storage space as a metaphor for a variable. You can rent a space (declaring the variable), and when you do so, you can begin to place things in that space (assigning data to the variable). At a later time, you may want to view the things from the storage space (retrieving the value from the variable), or you may decide to place other contents in the storage space (assigning a new value to the same variable). And when you are done with the storage space, you can stop renting it (deleting the variable).
Declaring Variables
Before you can meaningfully use a variable, you must first bring it into being by declaring it. Flash 8 and ActionScript 2.0 let you declare variables using strong typing. Strong typing means that when you create the variable, you also specify the type of data that it can hold. When you export your movie, Flash then makes sure that you consistently tried to store the correct type of data in that variable. If Flash detects that you mismatched the datatype with the variable at any point, an error message is generated, alerting you to the fact. This is helpful
for ensuring that your Flash applications are well planned and designed using good coding practices.
To declare a variable using strong typing, use the following pattern:
var variableName:Datatype;
The var keyword lets Flash know that you are declaring a variable. The variable name is up to your choosing, but it should follow the rules for variable naming (see the next section, ―Naming Variables‖). A colon separates the name of the variable and the name of the datatype, and there should be no space between the name, colon, or datatype. If you have code hinting turned on, you should get a drop-down list of built-in datatypes from which you can select. Alternatively, if you have defined your own custom datatype (see Chapter 5), you can declare a variable of that type as well. You‘ll also see that the line en ds with a semicolon.
Here is an example in which you declare a variable named nQuantity as a number:
var nQuantity:Number;
Now, you‘ve declared a variable with the name nQuantity, and you‘ve told Flash that all values you assign to the variable must be numbers. The variable has been created, but you have not defined any value for it yet. If you use trace() to display the value of the variable, you can see that this is so:
trace(nQuantity);
When you test this, you should see the value undefined appear in the Output panel. The value undefined is a special value that Flash uses for any variable that has not yet been assigned any value.
Once a variable has been declared, you can assign a value to it using a simple assignment statement with an equals sign:
var nQuantity:Number;
nQuantity = 6;
You can also declare the variable and assign a value, or initialize the variable, all on one line:
var nQuantity:Number = 6;
In addition to the undefined value, there is another special value, null, that you can assign to a variable that indicates that the variable does not contain any other, specific value. While undefined is used to indicate no value has been assigned to a variable, you can use null to indicate that a variable has intentionally been left without any other value. It is often a good practice to initialize your variables to some value other than undefined. And because null allows you to quickly distinguish between values intentionally or unintentionally left without another value, it is a good practice to initia lize your variables to null when you don‘t have any
other specific value to assign to them:
var nQuantity:Number = null;
You can use a variable in any situation in which you could use the value the variable contains. You‘ve already seen an example of this with the trace() actions. You can use a variable to tell Flash what message to output:
var sMessage:String = “Welcome!”;
trace(sMessage);
You can also perform other kinds of operations using variables, just as you would on the actual values themselves. For example:
var nQuantity:Number = 6;
var nPrice:Number = 9.99;
trace(nQuantity * nPrice); // Displays: 59.94
Naming Variables
Now that you‘ve looked at declaring and defining variables, the next thing to examine is how to name the variables. There are two main parts to this discussion. First, there is the matter of using valid variable names that Flash will understand, so we‘ll look at the rules for naming variables. Second, we‘ll examine some additional guidelines for naming variables that, while not strictly enforced by Flash, will aid you in creating more readable code.
The following are the rules that you must follow for Flash to be able to understand your variable names:
◆The first character must be an underscore (_), a dollar sign ($), or a letter. The first
character cannot be a number. Although underscores and dollar signs are allowable as the first character, in practical application, you will almost always start the variable name with a letter.
◆The subsequent characters must be underscores (_), dollar signs ($), letters, or
numbers.
◆Variable names can have no spaces.
◆The name cannot be a keyword or other special value recognized by Flash. For
example, the names MovieClip, true, String, and undefined are not allowable variable names because they already have other meanings in ActionScript.
◆The name must be unique (within its scope). If you create two variables with the same
name in the same scope (more on scope in Chapters 4 and 5), the latter will overwrite the former.
Next, let‘s talk about some good n aming conventions that you can use. First, because you‘ll want to be using strong typing with all your variables, it is a good idea to have a
convenient way to be reminded of what type of value a variable can hold. A system named Hungarian notation has bee n devised that can assist in this. For our purposes, we‘ll use a modification of Hungarian notation specifically designed for ActionScript. With this system, you can prefix each variable name with a character (or, in some cases, several characters) that can help you to remember what type of datatype the variable can hold. You may have already seen this in the previous examples in this chapter. When we define a variable named nQuantity, the variable name is prefixed with the character n. This tells us that the variable holds a number value. Table 3-1 shows a list of other recommended prefixes.
Table 3-1: Modified Hungarian Notation ActionScript Prefixes for Common Classes Prefix Datatype
a Arra y
bmp BitmapData
b Boolean
bt Button
c Color
cam Camera
cm ContextMenu
cmi ContextMenuItem
d Date
lc LocalConnection
lv LoadVars
m MovieClip
mcl MovieClipLoader
mic Microphone
n Number
nc NetConnection
ns NetStream
o Object
pj PrintJob
rs RecordSet
s String
snd Sound
so SharedObject
t TextField
tf TextFormat
vid Video
xml XML
xmls XMLSocket
This modified Hungarian notation convention is completely optional, but it can be very useful. It helps not only you, but also others who may read your code. By adding the appropriate prefix to the variable name, it makes it immediately clear what type of datatype the variable can hold.
It‘s also important when naming your variables to make the names as descriptive as possible. For example, the variable name nQuantity is much more descriptive than nQ. Of course, the level of descriptiveness required depends on the context. For example, if your Flash application deals with quantities of widgets as well as cogs, nQuantity might not be sufficiently clear. It would be better, in such a case, to have variables named nQuantityCog and nQuantityWidget, for example. The more descriptive the variable name, the better, in most situations. Just remember, though, that most likely you‘ll be typing the same variable name multiple times, so it is important to achieve the correct balance between descriptiveness and name length. You can always use abbreviations in the variable names if appropriate. For example, rather than defining a variable named nQuantityWidget you might find it easier to define a variable named nQntyWidget.
Remember that you cannot use spaces in your variable names. However, when you want to make your variable names descriptive, the names will often consist of more than one word. There are two conventions that are commonly used when naming variables with multiple words. The first of the two conventions is to use the underscore (_) to separate your words in the variable name. An example of this method follows:
var sFirst_name:String = “Joey”;
The second of these conventions is what is known as the interCap method (also known as studlyCaps or camelCaps). The word ―interCap‖ refers to the capitalization of the first letter of each word subsequent to the first, using no spaces or underscores—internal capitalization. An example of this method is the following:
var sFirstName:String = “Joey”;
It would behoove you to use one of these conventions. Pick one that you like, and if you decide you prefer the other one later on, switch to it. In this book we tend to prefer the interCap method, so you see a preference for it in the examples. But neither convention is more correct or offers any advantages over the other one.
It is also important to remember that ActionScript is case-sensitive. This means that sFirstName, sFirstname, SfirstName, and so on are all different variables. If you accidentally
type the name of a variable with incorrect capitalization, the result will be that Flash will not recognize that the variable is defined. Here is an example:
var sFirstName:String = “Joey”;
trace(sFirstName); // Displays: Joey
trace(sfirstName); // Displays: undefined
Using Expressions
Anyone who has taken even very basic arithmetic (using addition and subtraction and the like) has worked with expressions. Expressions are simply those parts of statements that evaluate to be equal to something. Here are some very simple examples:
1
“abcd”
nQuantity
Even though these examples are all either simple values or variables, they all evaluate to a single value, and therefore, are considered expressions. Slightly more complex (although still simple) expressions might look something like this:
1 + 1
“a”+ “b”
nQuantity * nPrice
Expressions are an important part of ActionScript. You use expressions in all kinds of situations.
For example, you can use an expression within a trace() statement:
trace(“Welcome!”);
You can also use expressions in variable assignment statements:
var nQuantity:Number = 6;
var nPrice:Number = 9.99;
var nTotal:Number = nQuantity * nPrice;
We‘ll also look at other kinds of expressions that perform comparisons and tests. F or example:
6 < 10
55 == 56
In the preceding examples, we use comparison operators to determine how two values compare. The first expression is determining whether 6 is less than 10. That expression evaluates to true. The second expression determines whether 55 is equal to 56. That expression evaluates to false.
There are many other examples of expressions, and we‘ll examine some of them
throughout the rest of this chapter.
Working with Operators
As you have seen, expressions can be composed of a single value. But expressions can also be more complex by combining several different values to make one expression. In expressions involving multiple values, there are two types of ActionScript elements involved: operands and operators. The operands are the values on which the operation acts. The operators determine the action taken. In the expression 4 + 5, for instance, there are two values, or operands — 4 and 5 — and there is one operator: the plus operator (+).
In the Actions toolbox you can see that the operators are grouped into six categories: arithmetic, assignment, bitwise, comparison, logical, and miscellaneous. The following sections take a closer look at each of these groups, and the operators of which they are composed. We‘ll look at each of these groups in the order they are listed in the Actions toolbox, with the exception of the bitwise operators. We‘ll look at the bitwise operators after the rest because, except in specialized situations, you are more likely to use the other operators than you are to use bitwise operators.
Working with Arithmetic Operators
The arithmetic operators should be familiar to you because they are, for the most part, the operators you used in math class. They are the operators used on number operands for mathematical computations. The result of an operation using an arithmetic operator is a number value. Table 3-2 lists all the arithmetic operators.
Table 3-2: Arithmetic Operators
Operator Name Example Result + Plus x + y x + y
- Minus/Negation x –y
-x x –yx * (-1)
* Multiply x * y x * y
/ Divide x / y x / y
% Modulo x % y Remainder of x / y
The addition, subtraction, multiplication, and division operators don‘t really require any discussion.They work just as you would expect them to.
The modulo operator (%) may be new to you. Even if it is not new, you might need a little refresher on what it does. Quite simply, it returns the value of the remainder after the first operand is divided by the second. In the following example, we use the modulo operator with a variable nYear and the second operand of 4. The result is 0. This means that 2004 is divisible by 4. In practical terms, the implication of this is that the year 2004 is a leap year.
var nYear:Number = 2004;
trace(nYear % 4); // Displays: 0
Also worth pointing out is that the minus and negation operators use the same character, but operate differently. The negation operator has the same effect as multiplying a number by –1. For example, this operation:
y = -x;
is the same as this operation:
y = x * (-1);
Working with Assignment Operators
Table 3-3 presents a rather daunting list of operators that all fall under the category of assignment operators. But don‘t be scared off just yet. In fact, there is only one fundamental operator in the bunch—the equals sign (=). This one should not be a new operator to you. It does just as you would expect it to do: It assigns the value of the operand on the right to the operand on the left. The remainder of the operators are compound assignment operators that function as shortcuts, as you‘ll see in a moment.
In the following example, the operand on the left (nQuantity) is assigned the value of the operand on the right (6):
nQuantity = 6;
Of course, you might want to use expressions that are slightly more complex than simply nQuantity = 6. You might, for instance, want to add several operands together on the right side of the equals sign operator, as in the following:
nQuantity = 6 + 36 + 24;
In this case, you notice that the addition takes place before the assignment. In other words, nQuantity is assigned the value of the sum of 6, 36, and 24, not just the value of 6. This is due to the operator precedence of the plus operator (+) being greater than that of the assignment operator (=). See the section later in this chapter titled ―Cons idering Operator Precedence.‖ Additionally, you can find a complete list of operators and their precedence in the Flash Help system.
Table 3-3: Assignment Operators
Operator Name Example What It Means = Equals(assignment) x = y x = y
+= Add by value x += y x = x + y
-= Subtract by value x -= y x = x - y
*= Multiply by value x *= y x= x * y
/= Divide by value x /= y x = x / y
%= Modulo by value x %= y x = x % y
<<= Left shift by value x <<= y x = x << y
>>= Right shift by value x >>= y x = x >> y >>>= Right shift zero fill by value x >>>= y x = x >>> y &= Bitwise AND by value x &= y x = x & y
|= Bitwise OR by value x |= y x = x | y
^= Bitwise XOR by value x ^= y x = x ^ y
As mentioned, there is really only one fundamental operator in the assignment operator category —the equals sign. Each of the additional operators merely saves you some time typing. For example, the following expression:
nQuantity += 6;
is the shorthand version of the following:
nQuantity = nQuantity + 6;
Either of the two preceding expressions means that you want Flash to add 6 to the current value of nQuantity. It just so happens that the former variation is shorter and quicker to type.
The operators that are compounded with the equals (=) operator are either mathematical operators or bitwise operators (covered in the following sections of this chapter). In each case, the compound operator follows the same pattern. For example:
nQuantity *= 6;
is the same as:
nQuantity = nQuantity * 6;
Working with Comparison Operators
Comparison operators allow you to compare operands. The result of a comparison is a Boolean value: true or false. These operators are most often used in expressions called conditionals within if...else blocks, and control of flow expressions within for and while blocks. (You learn about these types of statements later in this chapter.) But the basic premise is that if the conditional expression evaluates to true, a block of code is executed, and if it evaluates to false, the block of code is skipped over.
Using comparison operators (see Table 3-4), you can compare strings, numbers, and Boolean values. These primitive datatypes are equal only if they contain the same value. You can also compare reference data types such as objects, functions, and arrays. But reference data types are equal only if they reference the same object, whether or not they contain the same value.
Table 3-4: Comparison Operators
Operator Name
== Equals
!= Not equals
> Greater than
< Less than
>= Greater than or equal
<= Less than or equal
=== Strict equality
!== Strict inequality Perhaps the most common mistake made in programming is confusing the equality equals operator (==) with the assignment equals operator (=). In fact, even among seasoned professionals, it is not uncommon to make this error on occasion. The difference is so tiny in print, but the result is so drastic. Take, for instance, the following example:
var nQuantity:Number = 999;
if (nQuantity = 4){
trace(“if condition true”);
}
trace(nQuantity);
What would you expect th is code to do? Even if you don‘t know what some of the code structures mean, you can probably figure out that after nQuantity is assigned a value of 999, you want the code to check to see if nQuantity is equal to 4. If that condition is true, it should write a message to the Output panel. Finally, it writes the value of nQuantity to the Output panel.
You might expect that the final value of nQuantity is still 999. But because the wrong operator was mistakenly used in the if condition (nQuantity = 4), nQuantity has been assigned the value of 4! Can you see the problems that have been caused by one missing character? So, the corrected code looks more like this:
var nQuantity:Number = 999;
if (nQuantity == 4){
trace(“if condition true”);
}
trace(nQuantity);
Any datatype can be compared using the equality operators. String characters are first converted to the ASCII values and then compared, character by character. Therefore, ―a‖is less than ―z‖ and lowercase letters have higher values than their uppercase count erparts. Tables 5-5 and 5-6 show examples of numbers and strings being compared, using the equality
operators along with the resulting value of the expression.
Table 3-5: Number Comparison
Expression Result
6 == 6 true
6 != 6 false
6 > 6 false
6 < 6 false
6 >= 6 true
6 <= 6 true
Table 3-6: String Comparison
Expression Result
“Joey”== “Joey”true
“joey”!= “Joey”true
“joey”> “Joey”true
“Joey”< “Joseph”true
The only two operators in this category that you have not yet looked at are the strict equality (===) and strict inequality (!==) operators. These operators work much like the nonstrict counterparts (== and !=) with one difference: They don‘t perform datatype conversions automatically. What this means is that when using the regular equality equals operator (==), Flash automatically converts the operands to the same datatype before testing for equality. Therefore, the values 5 and “5”are equal when testing using the regular equality operator (==) but not when using the strict equality operator (===). Table 3-7 gives some examples of the difference between using regular and strict equality operators.
Table 3-7: Strict Equality and Inequality Operators
Regular Regular Result Strict Strict Result
6==6 true 6===6 true
6!=6 false 6!==6 false 6==”6”true 6===”6”false
6!=”6”false 6!==”6”true
译文:Flash 8 ActionScript 宝典,[美]Joey Lott, Robert Reinhaedt著,Wiley 出版社
Flash 8 ActionScript 宝典
Joey Lott, Robert Reinhardt
了解数据类型
只要谈到数据,就会谈到值。这些值可以有许多类型。例如,即使在一个非常简单的影片中,也会有一个数字、一些文本和一个MovieClip实例;它们都是具有不同类型(在ActionScript中称之为数据类型)的数据。
实际上,Flash可以在需要的时候执行数据类型转换。但是,这可能会使Acti。nScript 开发者养成某些拙劣的编码习惯。因此,ActionScript 2.0标准要求对正在使用的数据类型给予足够的注意。
在ActionScript中,将使用许多不同的数据类型。但是,为了了解这些数据类型如何工作,可以认为它们有两种基本的分类——简单类型和引用类型。简单数据类型之所以被称之为‖简单‖类型,是因为它们是基础的数据类型,而不是因为它们缺乏重要性。引用数据类型之所以被称之为‖引用‖类型,是因为它们引用简单类型。
简单数据类型包括字符串(String)、数字(Number)、布尔型(Boolean)、undefined和null。我们将在本章中更多地介绍这些简单数据类型。引用数据类型就是所有的对象,它们是本书其他章节的主题,因此,我们将对引用数据类型的主要讨论留到后面的章节中。
使用字符串数据
字符串是多个字符或多个宇。字符串的值必须用单引号或双引号引起来。下面是几个字符串的例子:
―a‖
?b‘
―l‖
―Joey‖
?123‘
?abc‘
―****‖
只要想使用多个字符或多个宇,就要使用字符串。例如,可以使用字符串来填充影片中的文本区域,或者使用字符串通过程序给新的MovieClip实例创建一个名字。我们已经见过如何在动作中使用字符串了,如trace()中的字符串。Trace()动作要求开发者给它提供一条在Output面板中显示的消息,这条消息就必须是字符串值:trace (―I kn o w him; Marley‘s Gh o st!‖);
如前所述,当定义一个字符串值时,可以使用双引号或单引号。究竟选择哪一个常
常完全是由个人爱好所决定的。但是,如果想让代码不出错误,有两个规则还是必须遵守的:第一,必须对每个开始的引号匹配一个结束的引号。第二,使用哪种类型的引号来开始字符串,就必须用哪种类型的引号来结束字符串。换句话说,不允许有不匹配的引号。下面是两个正确匹配了引号的例子:
―here is a string‖
?here is a string‘
下面是三个没有正确匹配引号的例子:
―here is a string‘
?here is a string‖
―here is a string
有些时候,更多的个人爱好可能会限制选择使用哪种类型的引号。试一试下面的例子,请注意会发生的事情:
trace ( ?I kn o w him; Marley‘s Gh o st !‘) ;
这行代码实际上会产生一个错误,因为它认为撇号是结束的引号,所以它就不知道用这个字符串的剩余部分做什么了。如果在字符串两边使用双引号,就可以很容易地修改这个错误了:
trace (―I kno w him; Marley‘s Gh ost!¨);
反之亦然。如果想在一个字符串中将一个双引号标记当做一个字符来用,可以在整个值的两边使用单引号。如果想在一个字符串中将单引号和双引号标记两者都当做字符来用,问题就来了。有一个简单的方法来处理这个问题:使用特殊字符。有关特殊字符的详细内容,请参见第1 1章。
使用数字数据
在Flash中,所有的数字都被当做“数字”数据类型。正数、负数、浮点数、整数等,都被简单地认为是数字,这通常不需要做进一步的解释。
为了定义一个数字,只需要输入不带任何引号的数字即可。下面是几个数字的例子:6
12
-1
3.3
1. 8
数字数据类型可以执行各种数学运算,如下面的例子所示:
trace(5 + 5); //显示10
trace(5 – 4); //显示1
trace(5 / 2); //显示2.5
了解什么时候使用数字而什么时候使用字符串是重要的。假如在实际上想用一个数
字的时候却用了一个字符串,就会得到不希望的结果。例如
trace ("5" + "5") ; //显示55
在这个例子中,结果值是“55”而不是10(所希望的)。这是因为ActionScript将这两个值当做字符串并将它们“连接”起来,而不是当做数字值将它们加起来。
当然,还应该了解,把数字当做字符串也有些合理的原因o例如,尽管电话号码是由数字组成的,但通常还是把它当做字符串o如果想用空格、括号、破折号等来对电话号码进行格式设置,就需要将电话号码放入引号中当成字符串来用。
使用布尔数据
布尔数据是只能取true(真)和false(假)两个值的数据。在Flash影片中,布尔变量常用于测试条件。布尔值常用于条件语句(如if语句)和控制结构(如for和while 语句)的条件表达式中。有关条件语句和控制结构的详细内容,请参见本章后面的“使用控制流程的语句——控制结构”一节。
理解undefined和null数据类型
ActionScript有两个另外的基本数据类型——undefined(未定义)和null(空值)。开发者应该熟悉它们。为了更好地理解这些数据类型,先熟悉变量是有用的。因此,我们将在本章的后面交替地讨论这两种数据类型和变量。
转换数据
通过被称为“转换( Casting)”的方法,ActionScript允许你告诉Flash将一个值转换成一个指定的数据类型。当转换一个值时,使用如下语法:
Datatype(value)
例如,可以像下面这样将一个字符串转换成数字:
Number(―123‖)
当转换数据时必须小心。某些转换可能会产生不希望的结果。例如,数宇0可以被转换成布尔值false,任何非零值将被转换成true,例如:
trace (Boolean (0) ) : //显示false
trace (Boolean (1) ) ; //显示true
因此,如果转换true和false的字符串值,它们两者都将被转换成布尔值true,因为这两个字
符串的值都是非零值:
氛参使用变量
trace (Boolean (―true‖) ) ; //显示true
trace (Boolean (―flase‖) ) ; //显示true
使用变量
在Flash影片中,将会用到许多数据。现在,由于这些数据到处都是,所以我们打算用某种办法来跟踪它们。这就是变量变得有用的原因。
变量是一个可以用来保存或引用某个特殊数据的命名了的容器。一旦创建了一个变量,就可以在该变量中保存和取回数据。尽管一个变量一次只能容纳一个值,但可以使用同一个变量在不同的时候容纳或引用不同的数据。例如,如果创建了一个名为nYear 的变量,它在一个时候可以容纳2005这个值,但在另一个时候可以容纳2006这个值。
用一个出租仓库空间来比喻一个交量。可以租一个空间(声明变量),租了之后,就可以在那个空间放置东西了(给变量赋值)。后来,可能想看一下仓库空间中放置的东西(从变量中取回数据),或者可能决定在该仓库空间中放置别的东西(给同一个变量赋予一个新值)。当用这块仓库空间办完事后,可以不再租用它(删除该变量)。
声明变量
在能有意识地使用一个变量之前,必须首先声明它以使其存在。Flash 8和ActionScript 2.0允许使用强化输入法来声明变量o强化输入法表示,当创建一个变量时,指定它可以保存的数据类型。当导出影片时,Flash就确保用户始终想向那个变量中存入正确数据类型的数据。如果Flash在任何时候发现没能匹配该变量的数据类型,就会产生一条出错消息,提醒用户注意这个实际情况。这有助于确保Flash应用程序是用良好的编程习惯进行周密的规划和设计的。
为了用强化输入法来声明变量,请使用如下的格式:
var variableName:Datotype ;
var关键宇让Flash知道正在声明一个变量。变量名由用户取,但它应该遵守变量的命名规则(参见下一节——“命名变量”)。用冒号来分隔变量的名字和数据类型的名字-在名字、冒号、数椐类型之间不应该有空格。如果启用了代码提示,就会获得一个内置数据类型的下拉列表,可以从该下拉列表中选择数据类犁。另外,如果定义过自己的自定义数据类型(参见第5章),也可以声明一个那种数据类型的变量。还可以看到,该行是用一个分号来结束的。
下面的例子声明了一个名为nQuantity的数字数据类型的变量:
var nQuantity : Number ;
现在,已经声明了一个名为nQuantity的变量,并且已经告诉Flash,赋予该变量的所有值都必须是数字。变量已经被创建了,但还没有给它定义任何值。如果用trace()来显示该变量的值,就可以知道的确是这样:
trace(nQuantity) ;
当对它进行测试时,会见到undefined值出现在Output面板中。undefined值是一个特殊的值,Flash用它来表示还没有被赋予任何值的变量。
变量一旦被声明了,就可以通过使用一个具有等于符号的简单赋值语句来给它赋值:var nQuantity :Number ;
nQuantity = 6 ;
还可以在一行中声明一个变量并对其赋值,或初始化该变量
var nQuantity : Number = 6;
除了undefined值,还有另一个特殊的值——null,可以将它赋予一个变量,表示该变量没有容纳任何其他指定的值。undefined值常用于表示还没有给一个变量赋值,null值表示一个变量已经被有意留下束而没有任何其他值。将变置初始化为不是undefined的某个值常常是一个好的习惯。因为null可以使用户迅速地区分有意或无意留下来、没有其他值的变量,所以当没有任何其他指定的值要赋予变量时,将变量初始化为null常常是一个好的习惯:
var nQuantity : Number = null ;
可以在能使用一个变量所容纳的值的任何地方使用该变量o我们已经在trace()动作中看见了这样的一个例子。可以用一个变量来告诉Flash要输出什么消息:var sMessage : String = ―Welcome !‖ ;
trace (sMessage) ;
还可以使用变量来执行其他种类的操作,就像使用实际值那样。例如:
var nQuantity : Number = 6 ;
var nPrice : Number = 9.99 ;
trace (nQuantity * nPrice) ; //显示59.94
命名变量
现在已经知道声明和定义变量了,下一步要做的事情就是命名变量。这个问题要分成两个主要部分来讨论。第一,要注意使用Flash理解的、有效的变量名字。因此,我们要探讨命名变量的规则。第二,我们将考察某些用于命名变量的其他指导方针,Flash 并不强求这样,但它们将有助于刨建更可读的代码。
为了让Flash能够理解变量,必须遵守如下规则:
◆第一个字符必须是下划线(_ )、美元符号($ )或字母。第一个字符不能是
数字。尽管可以用下划线和美元符号来作为第一个字符,但在实际应用中,通
常应该用一个字母来开始一个变量名。
◆接下来的字符必须是下划线(_ )、美元符号($ )、字母或数字。
◆在变量名中不能有空格。
◆名字不能是关键宇或其他被Flash识别的特殊的值。例如,MovieClip,true,String,
undefined就是不被允许的变量名,因为它们在ActionScript中已经有其他意义
了。
◆名字必须是惟一的(在它的作用域中)。如果在同一个作用域(有关作用域的详
细内容,请参见第4章和第5章)中创建了两个名字相同的变量,那么后面的
一个就将覆盖前面的一个。
接下来,让我们介绍一些好的、可以用的命名习惯。第一,因为希望在所有变量中都使用强化输入法,所以要是有一个方便的方法来提醒一个变量可以保存什么类型的值
PLC控制下的电梯系统外文文献翻译、中英文翻译、外文翻译
PLC控制下的电梯系统 由继电器组成的顺序控制系统是最早的一种实现电梯控制的方法。但是,进入九十年代,随着科学技术的发展和计算机技术的广泛应用,人们对电梯的安全性、可靠性的要求越来越高,继电器控制的弱点就越来越明显。 电梯继电器控制系统故障率高,大大降低了电梯的可靠性和安全性,经常造成停梯,给乘用人员带来不便和惊忧。且电梯一旦发生冲顶或蹲底,不但会造成电梯机械部件损坏,还可能出现人身事故。 可编程序控制器(PLC)最早是根据顺序逻辑控制的需要而发展起来的,是专门为工业环境应用而设计的数字运算操作的电子装置。鉴于其种种优点,目前,电梯的继电器控制方式己逐渐被PLC控制所代替。同时,由于电机交流变频调速技术的发展,电梯的拖动方式己由原来直流调速逐渐过渡到了交流变频调速。因此,PLC控制技术加变频调速技术己成为现代电梯行业的一个热点。 1. PLC控制电梯的优点 (1)在电梯控制中采用了PLC,用软件实现对电梯运行的自动控制,可靠性大大提高。 (2)去掉了选层器及大部分继电器,控制系统结构简单,外部线路简化。 (3)PLC可实现各种复杂的控制系统,方便地增加或改变控制功能。 (4) PLC可进行故障自动检测与报警显示,提高运行安全性,并便于检修。 (5)用于群控调配和管理,并提高电梯运行效率。 (6)更改控制方案时不需改动硬件接线。 2.电梯变频调速控制的特点 随着电力电子技术、微电子技术和计算机控制技术的飞速发展,交流变频调速技术的发展也十分迅速。电动机交流变频调速技术是当今节电、改善工艺流程以提高产品质量和改善环境、推动技术进步的一种主要手段。变频调速以其优异的调速性能和起制动平稳性能、高效率、高功率因数和节电效果,广泛的适用范围及其它许多优点而被国内外公认为最有发展前途的调速方式 交流变频调速电梯的特点 ⑴能源消耗低 ⑵电路负载低,所需紧急供电装置小 在加速阶段,所需起动电流小于2.5倍的额定电流。且起动电流峰值时间短。由于起动电流大幅度减小,故功耗和供电缆线直径可减小很多。所需的紧急供电
电子信息工程外文翻译外文文献英文文献微处理器
外文资料 所译外文资料: 1. 作者G..Bouwhuis, J.Braat, A.Huijser 2. 书名:Principles of Optical Disk Systems 3. 出版时间:1991年9月 4. 所译章节:Session 2/Chapter9, Session 2/Chapter 11 原文: Microprocessor One of the key inventions in the history of electronics, and in fact one of the most important inventions ever period, was the transistor. As time progressed after the inven ti on of LSI in tegrated circuits, the tech no logy improved and chips became smaller, faster and cheaper. The functions performed by a processor were impleme nted using several differe nt logic chips. In tel was the first compa ny to in corporate all of these logic comp onents into a si ngle chip, this was the first microprocessor. A microprocessor is a complete computati on engine that is fabricated on a sin gle chip. A microprocessor executes a collecti on of machi ne in struct ions that tell the processor what to do. Based on the in struct ions, a microprocessor does three basic things: https://www.360docs.net/doc/7b13056157.html,ing the ALU (Arithmetic/Logic Unit), a microprocessor can perform mathematical operatio ns like additi on, subtract ion, multiplicatio n and divisi on; 2.A microprocessor can move data from one memory location to another; 3.A microprocessor can make decisi ons and jump to a new set of in struct ions based on those decisi ons. There may be very sophisticated things that a microprocessor does, but those are its three basic activities. Microprocessor has an address bus that sends an address to memory, a data bus that can send data to memory or receive data from memory, an RD(read) and WR(write) line that lets a clock pulse sequenee the processor and a reset li ne that resets the program coun ter to zero(or whatever) and restarts executi on. And let ' s assume that both the address and data buses are 8 bits wide here. Here are the comp onents of this simple microprocessor: 1. Registers A, B and C are simply latches made out of flip-flops. 2. The address latch is just like registers A, B and C. 3. The program coun ter is a latch with the extra ability to in creme nt by 1 whe n told to do so, and also to reset to zero whe n told to do so. 4. The ALU could be as simple as an 8-bit adder, or it might be able to add, subtract, multiply and divide 8- bit values. Let ' s assume the latter here. 5. The test register is a special latch that can hold values from comparisons performed in the ALU. An ALU can normally compare two numbers send determine if they are equal, if one is greater
土木工程外文翻译
转型衰退时期的土木工程研究 Sergios Lambropoulosa[1], John-Paris Pantouvakisb, Marina Marinellic 摘要 最近的全球经济和金融危机导致许多国家的经济陷入衰退,特别是在欧盟的周边。这些国家目前面临的民用建筑基础设施的公共投资和私人投资显著收缩,导致在民事特别是在民用建筑方向的失业。因此,在所有国家在经济衰退的专业发展对于土木工程应届毕业生来说是努力和资历的不相称的研究,因为他们很少有机会在实践中积累经验和知识,这些逐渐成为过时的经验和知识。在这种情况下,对于技术性大学在国家经济衰退的计划和实施的土木工程研究大纲的一个实质性的改革势在必行。目的是使毕业生拓宽他们的专业活动的范围,提高他们的就业能力。 在本文中,提出了土木工程研究课程的不断扩大,特别是在发展的光毕业生的潜在的项目,计划和投资组合管理。在这个方向上,一个全面的文献回顾,包括ASCE体为第二十一世纪,IPMA的能力的基础知识,建议在其他:显著增加所提供的模块和项目管理在战略管理中添加新的模块,领导行为,配送管理,组织和环境等;提供足够的专业训练五年的大学的研究;并由专业机构促进应届大学生认证。建议通过改革教学大纲为土木工程研究目前由国家技术提供了例证雅典大学。 1引言 土木工程研究(CES)蓬勃发展,是在第二次世界大战后。土木工程师的出现最初是由重建被摧毁的巨大需求所致,目的是更多和更好的社会追求。但是很快,这种演变一个长期的趋势,因为政府为了努力实现经济发展,采取了全世界的凯恩斯主义的理论,即公共基础设施投资作为动力。首先积极的结果导致公民为了更好的生活条件(住房,旅游等)和增加私人投资基础设施而创造机会。这些现象再国家的发展中尤为为明显。虽然前景并不明朗(例如,世界石油危机在70年代),在80年代领先的国家采用新自由主义经济的方法(如里根经济政策),这是最近的金融危机及金融危机造成的后果(即收缩的基础设施投资,在技术部门的高失业率),消除发展前途无限的误区。 技术教育的大学所认可的大量研究土木工程部。旧学校拓展专业并且新的学校建成,并招收许多学生。由于高的职业声望,薪酬,吸引高质量的学校的学生。在工程量的增加和科学技术的发展,导致到极强的专业性,无论是在研究还是工作当中。结构工程师,液压工程师,交通工程师等,都属于土木工程。试图在不同的国家采用专业性的权利,不同的解决方案,,从一个统一的大学学历和广泛的专业化的一般职业许可证。这个问题在许多其他行业成为关键。国际专业协会的专家和机构所确定的国家性检查机构,经过考试后,他们证明不仅是行业的新来者,而且专家通过时间来确定进展情况。尽管在很多情况下,这些证书虽然没有国家接受,他们赞赏和公认的世界。 在试图改革大学研究(不仅在土木工程)更接近市场需求的过程中,欧盟确定了1999博洛尼亚宣言,它引入了一个二能级系统。第一级度(例如,一个三年的学士)是进入
电子技术专业英语翻译
基本电路 包括电路模型的元素被称为理想的电路元件。一个理想的电路元件是一个实际的电气元件的数学模型,就像一个电池或一个灯泡。重要的是为理想电路元件在电路模型用来表示实际的电气元件的行为可接受程度的准确性。电路分析,本单位的重点,这些工具,然后应用电路。电路分析基础上的数学方法,是用来预测行为的电路模型和其理想的电路元件。一个所期望的行为之间的比较,从设计规范,和预测的行为,形成电路分析,可能会导致电路模型的改进和理想的电路元件。一旦期望和预测的行为是一致的,可以构建物理原型。 物理原型是一个实际的电气系统,修建从实际电器元件。测量技术是用来确定实际的物理系统,定量的行为。实际的行为相比,从设计规范的行为,从电路分析预测的行为。比较可能会导致在物理样机,电路模型,或两者的改进。最终,这个反复的过程,模型,组件和系统的不断完善,可能会产生较准确地符合设计规范的设计,从而满足需要。 从这样的描述,它是明确的,在设计过程中,电路分析中起着一个非常重要的作用。由于电路分析应用电路模型,执业的工程师尝试使用成熟的电路模型,使设计满足在第一次迭代的设计规范。在这个单元,我们使用20至100年已测试通过机型,你可以认为他们是成熟的。能力模型与实际电力系统理想的电路元件,使电路理论的工程师非常有用的。 说理想电路元件的互连可用于定量预测系统的行为,意味着我们可以用数学方程描述的互连。对于数学方程是有用的,我们必须写他们在衡量的数量方面。在电路的情况下,这些数量是电压和电流。电路分析的研究,包括了解其电压和电流和理解上的电压施加的限制,目前互连的理想元素的每一个理想的电路元件的行为电路分析基础上的电压和电流的变量。电压是每单位电荷,电荷分离所造成的断电和SI单位伏V = DW / DQ。电流是电荷的流动速度和具有的安培SI单位(I= DQ/ DT)。理想的基本电路元件是两个终端组成部分,不能细分,也可以在其终端电压和电流的数学描述。被动签署公约涉及元素,当电流通过元素的参考方向是整个元素的参考电压降的方向端子的电压和电流的表达式使用一个积极的迹象。 功率是单位时间内的能量和平等的端电压和电流的乘积;瓦SI单位。权力的代数符号解释如下: 如果P> 0,电源被传递到电路或电路元件。 如果p<0,权力正在从电路或电路元件中提取。 在这一章中介绍的电路元素是电压源,电流源和电阻器。理想电压源保持一个规定的电压,不论当前的设备。理想电流源保持规定的电流不管了整个设备的电压。电压和电流源是独立的,也就是说,不是任何其他电路的电流或电压的影响;或依赖,就是由一些电路中的电流或电压。一个电阻制约了它的电压和电流成正比彼此。有关的比例常数电压和一个电阻值称为其电阻和欧姆测量。 欧姆定律建立相称的电压和电流的电阻。具体来说,V = IR电阻的电流流动,如果在它两端的电压下降,或V=_IR方向,如果在该电阻的电流流是在它两端的电压上升方向。 通过结合对权力的方程,P = VI,欧姆定律,我们可以判断一个电阻吸收的功率:P = I2R= U2/ R 电路节点和封闭路径。节点是一个点,两个或两个以上的电路元件加入。当只有两个元素连接,形成一个节点,他们表示将在系列。一个闭合的路径是通过连接元件追溯到一个循环,起点和终点在同一节点,只有一次每遇到中间节点。 电路是说,要解决时,两端的电压,并在每个元素的电流已经确定。欧姆定律是一个重要的方程,得出这样的解决方案。 在简单的电路结构,欧姆定律是足以解决两端的电压,目前在每一个元素。然而,对于更复杂的互连,我们需要使用两个更为重要的代数关系,被称为基尔霍夫定律,来解决所有的电压和电流。 基尔霍夫电流定律是: 在电路中的任何一个节点电流的代数和等于零。 基尔霍夫电压定律是: 电路中的任何封闭路径上的电压的代数和等于零。 1.2电路分析技术 到目前为止,我们已经分析应用结合欧姆定律基尔霍夫定律电阻电路相对简单。所有的电路,我们可以使用这种方法,但因为他们而变得结构更为复杂,涉及到越来越多的元素,这种直接的方法很快成为累赘。在这一课中,我们介绍两个电路分析的强大的技术援助:在复杂的电路结构的分析节点电压的方法,并网电流的方
计算机专业外文文献及翻译
微软Visual Studio 1微软Visual Studio Visual Studio 是微软公司推出的开发环境,Visual Studio可以用来创建Windows平台下的Windows应用程序和网络应用程序,也可以用来创建网络服务、智能设备应用程序和Office 插件。Visual Studio是一个来自微软的集成开发环境IDE,它可以用来开发由微软视窗,视窗手机,Windows CE、.NET框架、.NET精简框架和微软的Silverlight支持的控制台和图形用户界面的应用程序以及Windows窗体应用程序,网站,Web应用程序和网络服务中的本地代码连同托管代码。 Visual Studio包含一个由智能感知和代码重构支持的代码编辑器。集成的调试工作既作为一个源代码级调试器又可以作为一台机器级调试器。其他内置工具包括一个窗体设计的GUI应用程序,网页设计师,类设计师,数据库架构设计师。它有几乎各个层面的插件增强功能,包括增加对支持源代码控制系统(如Subversion和Visual SourceSafe)并添加新的工具集设计和可视化编辑器,如特定于域的语言或用于其他方面的软件开发生命周期的工具(例如Team Foundation Server的客户端:团队资源管理器)。 Visual Studio支持不同的编程语言的服务方式的语言,它允许代码编辑器和调试器(在不同程度上)支持几乎所有的编程语言,提供了一个语言特定服务的存在。内置的语言中包括C/C + +中(通过Visual C++),https://www.360docs.net/doc/7b13056157.html,(通过Visual https://www.360docs.net/doc/7b13056157.html,),C#中(通过Visual C#)和F#(作为Visual Studio 2010),为支持其他语言,如M,Python,和Ruby等,可通过安装单独的语言服务。它也支持的 XML/XSLT,HTML/XHTML ,JavaScript和CSS.为特定用户提供服务的Visual Studio也是存在的:微软Visual Basic,Visual J#、Visual C#和Visual C++。 微软提供了“直通车”的Visual Studio 2010组件的Visual Basic和Visual C#和Visual C + +,和Visual Web Developer版本,不需任何费用。Visual Studio 2010、2008年和2005专业版,以及Visual Studio 2005的特定语言版本(Visual Basic、C++、C#、J#),通过微软的下载DreamSpark计划,对学生免费。 2架构 Visual Studio不支持任何编程语言,解决方案或工具本质。相反,它允许插入各种功能。特定的功能是作为一个VS压缩包的代码。安装时,这个功能可以从服务器得到。IDE提供三项服务:SVsSolution,它提供了能够列举的项目和解决方案; SVsUIShell,它提供了窗口和用户界面功能(包括标签,工具栏和工具窗口)和SVsShell,它处理VS压缩包的注册。此外,IDE还可以负责协调和服务之间实现通信。所有的编辑器,设计器,项目类型和其他工具都是VS压缩包存在。Visual Studio 使用COM访问VSPackage。在Visual Studio SDK中还包括了管理软件包框架(MPF),这是一套管理的允许在写的CLI兼容的语言的任何围绕COM的接口。然而,MPF并不提供所有的Visual Studio COM 功能。
伺服电机外文文献翻译
伺服电机 1. 伺服电机的定义 伺服电动机又称执行电动机,在自动控制系统中,用作执行元件,把所收到的电信号转换成电动机轴上的角位移或角速度输出。分为直流和交流伺服电动机两大类,其主要特点是,当信号电压为零时无自转现象,转速随着转矩的增加而匀速下降。伺服电机在伺服系统中控制机械元件运转的发动机. 是一种补助马达间接变速装置。伺服电机可使控制速度, 位置精度非常准确。将电压信号转化为转矩和转速以驱动控制对象。转子转速受输入信号控制,并能快速反应,在自动控制系统中作执行元件,且具有机电时间常数小、线性度高、始动电压低等特点。 2. 伺服电机工作原理 1.伺服主要靠脉冲来定位,基本上可以这样理解,伺服电机接收到1 个脉冲,就会旋转1 个脉冲对应的角度,从而实现位移,因为,伺服电机本身具备发出脉冲的功能,所以伺服电机每旋转一个角度,都会发出对应数量的脉冲,这样,和伺服电机接受的脉冲形成了呼应,或者叫闭环,如此一来,系统就会知道发了多少脉冲给伺服电机,同时又收了多少脉冲回来,这样,就能够很精确的控制电机的转动,从而实现精确的定位,可以达到0.001mm有刷电机成本低,结构简单,启动转矩大,调速范围宽,控制容易,需要维护,但维护方便(换碳刷),产生电磁干扰,对环境有要求。无刷电机体积小,重量轻,出力大,响应快,速度高,惯量小,转动平滑,力矩稳定。控制复杂,容易实现智能化,其电子换相方式灵活,可以方波换相或正弦波换相。电机免维护,效率很高,运行温度低,电磁辐射很小,长寿命,可用于各种环境。 2. 交流伺服电机也是无刷电机,分为同步和异步电机,目前运动控制中一般都用同步电机,它的功率范围大,可以做到很大的功率。大惯量,最高转动速度低,且随着功率增大而快速降低。因而适合做低速平稳运行的应用。 3. 永磁交流伺服电动机简介 20 世纪80 年代以来,随着集成电路、电力电子技术和交流可变速驱动技术的发展,永磁交流伺服驱动技术有了突出的发展,各国著名电气厂商相继推出各自的交流伺服电动机和伺服驱动器系列产品并不断完善和更新。交流伺服系统已成为当代高性能伺服系统的主要发展方向,使原来的直流伺服面临被淘汰的危机。90 年代以后,世界各国已经商品化了的交流伺服系统是采用全数字控制的正弦
电子信息工程专业课程翻译中英文对照表
电子信息工程专业课程名称中英文翻译对照 (2009级培养计划)
实践环节翻译 高等数学Advanced Mathematics
大学物理College Physics 线性代数Linear Algebra 复变函数与积分变换Functions of Complex Variable and Integral Transforms 概率论与随机过程Probability and Random Process 物理实验Experiments of College Physics 数理方程Equations of Mathematical Physics 电子信息工程概论Introduction to Electronic and Information Engineering 计算机应用基础Fundamentals of Computer Application 电路原理Principles of Circuit 模拟电子技术基础Fundamentals of Analog Electronics 数字电子技术基础Fundamentals of Digital Electronics C语言程序设计The C Programming Language 信息论基础Fundamentals of Information Theory 信号与线性系统Signals and Linear Systems 微机原理与接口技术Microcomputer Principles and Interface Technology 马克思主义基本原理Fundamentals of Marxism 思想、理论和“三个代表” 重要思想概论 Thoughts of Mao and Deng 中国近现代史纲要Modern Chinese History 思想道德修养与法律基 础 Moral Education & Law Basis 形势与政策Situation and Policy 英语College English 体育Physical Education 当代世界经济与政治Modern Global Economy and Politics 卫生健康教育Health Education 心理健康知识讲座Psychological Health Knowledge Lecture 公共艺术课程Public Arts 文献检索Literature Retrieval 军事理论Military Theory 普通话语音常识及训练Mandarin Knowledge and Training 大学生职业生涯策划 (就业指导) Career Planning (Guidance of Employment ) 专题学术讲座Optional Course Lecture 科技文献写作Sci-tech Document Writing 高频电子线路High-Frequency Electronic Circuits 通信原理Communications Theory 数字信号处理Digital Signal Processing 计算机网络Computer Networks 电磁场与微波技术Electromagnetic Field and Microwave Technology 现代通信技术Modern Communications Technology
土木工程外文翻译.doc
项目成本控制 一、引言 项目是企业形象的窗口和效益的源泉。随着市场竞争日趋激烈,工程质量、文明施工要求不断提高,材料价格波动起伏,以及其他种种不确定因素的影响,使得项目运作处于较为严峻的环境之中。由此可见项目的成本控制是贯穿在工程建设自招投标阶段直到竣工验收的全过程,它是企业全面成本管理的重要环节,必须在组织和控制措施上给于高度的重视,以期达到提高企业经济效益的目的。 二、概述 工程施工项目成本控制,指在项目成本在成本发生和形成过程中,对生产经营所消耗的人力资源、物资资源和费用开支,进行指导、监督、调节和限制,及时预防、发现和纠正偏差从而把各项费用控制在计划成本的预定目标之内,以达到保证企业生产经营效益的目的。 三、施工企业成本控制原则 施工企业的成本控制是以施工项目成本控制为中心,施工项目成本控制原则是企业成本管理的基础和核心,施工企业项目经理部在对项目施工过程进行成本控制时,必须遵循以下基本原则。 3.1 成本最低化原则。施工项目成本控制的根本目的,在于通过成本管理的各种手段,促进不断降低施工项目成本,以达到可能实现最低的目标成本的要求。在实行成本最低化原则时,应注意降低成本的可能性和合理的成本最低化。一方面挖掘各种降低成本的能力,使可能性变为现实;另一方面要从实际出发,制定通过主观努力可能达到合理的最低成本水平。 3.2 全面成本控制原则。全面成本管理是全企业、全员和全过程的管理,亦称“三全”管理。项目成本的全员控制有一个系统的实质性内容,包括各部门、各单位的责任网络和班组经济核算等等,应防止成本控制人人有责,人人不管。项目成本的全过程控制要求成本控制工作要随着项目施工进展的各个阶段连续 进行,既不能疏漏,又不能时紧时松,应使施工项目成本自始至终置于有效的控制之下。 3.3 动态控制原则。施工项目是一次性的,成本控制应强调项目的中间控制,即动态控制。因为施工准备阶段的成本控制只是根据施工组织设计的具体内容确
电力电子技术外文翻译
外文翻译 题目:电力电子技术二 A部分 晶闸管 在晶闸管的工作状态,电流从阳极流向阴极。在其关闭状态,晶闸管可以阻止正向 导电,使其不能运行。 可触发晶闸管能使导通状态的正向电流在短时间内使设备处于阻断状态。使正向电压下降到只有导通状态的几伏(通常为1至3伏电压依赖于阻断电压的速度)。 一旦设备开始进行,闸极电流将被隔离。晶闸管不可能被闸关闭,但是可以作为一个二极管。在电路的中,只有当电流处于消极状态,才能使晶闸管处于关闭状态,且电流降为零。在设备运行的时间内,允许闸在运行的控制状态直到器件在可控时间再次进入正向阻断状态。 在逆向偏置电压低于反向击穿电压时,晶闸管有微乎其微的漏电流。通常晶闸管的正向额定电压和反向阻断电压是相同的。晶闸管额定电流是在最大范围指定RMS和它是有能力进行平均电流。同样的对于二极管,晶闸管在分析变流器的结构中可以作为理想的设备。在一个阻性负载电路中的应用中,可以控制运行中的电流瞬间传至源电压的正半周期。当晶闸管尝试逆转源电压变为负值时,其理想化二极管电流立刻变成零。 然而,按照数据表中指定的晶闸管,其反向电流为零。在设备不运行的时间中,电流为零,重要的参数变也为零,这是转弯时间区间从零交叉电流电压的参
考。晶闸管必须保持在反向电压,只有在这个时间,设备才有能力阻止它不是处于正向电压导通状态。 如果一个正向电压应用于晶闸管的这段时间已过,设备可能因为过早地启动并有可能导致设备和电路损害。数据表指定晶闸管通过的反向电压在这段期间和超出这段时间外的一个指定的电压上升率。这段期间有时被称为晶闸管整流电路的周期。 根据使用要求,各种类型的晶闸管是可得到的。在除了电压和电流的额定率,转弯时间,和前方的电压降以及其他必须考虑的特性包括电流导通的上升率和在关闭状态的下降率。 1。控制晶闸管阶段。有时称为晶闸管转换器,这些都是用来要是整顿阶段,如为直流和交流电机驱动器和高压直流输电线路应用的电压和电流的驱动。主要设备要求是在大电压、电流导通状态或低通态压降中。这类型的晶闸管的生产晶圆直径到10厘米,其中平均电流目前大约是4000A,阻断电压为5之7KV。 2。逆变级的晶闸管。这些设计有小关断时间,除了低导通状态电压,虽然在设备导通状态电压值较小,可设定为2500V和1500A。他们的关断时间通常在几微秒范围到100μs之间,取决于其阻断电压的速率和通态压降。 3。光控晶闸管。这些会被一束脉冲光纤触发使其被引导到一个特殊的敏感的晶闸管地区。光化的晶闸管触发,是使用在适当波长的光的对硅产生多余的电子空穴。这些晶闸管的主要用途是应用在高电压,如高压直流系统,有许多晶闸管被应用在转换器阀门上。光控晶闸管已经发现的等级,有4kV的3kA,导通状态电压2V、光触发5毫瓦的功率要求。 还有其它一些晶闸管,如辅助型关断晶闸管(关贸总协定),这些晶闸管其他变化,不对称硅可控(ASCR)和反向进行,晶闸管(RCT)的。这些都是应用。 B部分 功率集成电路 功率集成电路的种类 现代半导体功率控制相当数量的电路驱动,除了电路功率器件本身。这些控制电路通常由微处理器控制,其中包括逻辑电路。这种在同一芯片上包含或作
计算机科学与技术设计论文外文翻译
本科毕业设计(论文)外文翻译(附外文原文) 系 ( 院 ):信息科学与工程学院 课题名称:学生信息管理系统 专业(方向):计算机科学与技术(应用)
7.1 Enter ActionMappings The Model 2 architecture (see chapter 1) encourages us to use servlets and Java- Server Pages in the same application. Under Model 2, we start by calling a servlet. The servlet handles the business logic and directs control to the appropriate page to complete the response. The web application deployment descriptor (web.xml) lets us map a URL pattern to a servlet. This can be a general pattern, like *.do, or a specific path, like saveRecord.do. Some applications implement Model 2 by mapping a servlet to each business operation. This approach works, but many applications involve dozens or hundreds of business operations. Since servlets are multithreaded, instantiating so manyservlets is not the best use of server resources. Servlets are designed to handle any number of parallel requests. There is no performance benefit in simply creating more and more servlets. The servlet’s primary job is to interact with the container and HTTP. Handling a business operation is something that a servlet could delegate to another component. Struts does this by having the ActionServlet delegate the business operation to an object. Using a servlet to receive a request and route it to a handler is known as the Front Controller pattern [Go3]. Of course, simply delegating the business operation to another component does not solve the problem of mapping URIs [W3C, URI] to business operations. Our only way of communicating with a web browser is through HTTP requests and URIs. Arranging for a URI to trigger a business operation is an essential part of developing a web application. Meanwhile, in practice many business operations are handled in similar ways. Since Java is multithreaded, we could get better use of our server resources if we could use the same Action object to handle similar operations. But for this to work, we might need to pass the object a set of configuration parameters to use with each operation. So what’s the bottom line? To implement Model 2 in an efficient and flexible way, we need to: Enter ActionMappings 195
双闭环直流调速系统外文翻译
对直流电机的速度闭环控制系统的设计 钟国梁 机械与汽车工程学院华南理工大学 中国,广州510640 电子邮件:zhgl2chl@https://www.360docs.net/doc/7b13056157.html, 机械与汽车工程学院 华南理工大学 中国,广州510640 江梁中 电子邮件:jianglzh88@https://www.360docs.net/doc/7b13056157.html, 该研究是由广州市科技攻关项目赞助(No.2004A10403006)。(赞助信息) 摘要 本文介绍了直流电机的速度控制原理,阐述了速度控制PIC16F877单片机作为主控元件,利用捕捉模块的特点,比较模块和在PIC16F877单片机模数转换模块将触发电路,并给出了程序流程图。系统具有许多优点,包括简单的结构,与主电路同步,稳定的移相和足够的移相范围,10000步控制的角度,对电动机的无级平滑控制,陡脉冲前沿,足够的振幅值,设定脉冲宽度,良好的稳定性和抗干扰性,并且成本低廉,这速度控制具有很好的实用价值,系统可以容易地实现。 关键词:单片机,直流电机的速度控制,控制电路,PI控制算法
1.简介 电力电子技术的迅速发展使直流电机的转速控制逐步从模拟转向数字,目前,广泛采用晶闸管直流调速传动系统设备(如可控硅晶闸管,SCR )在电力拖动控制系统对电机供电已经取代了笨重的F-D 发电机电动机系统,尤其是单片机技术的应用使速度控制直流电机技术进入一个新阶段。在直流调速系统中,有许多各种各样的控制电路。单片机具有高性能,体积小,速度快,优点很多,价格便宜和可靠的稳定性,广泛的应用和强劲的流通,它可以提高控制能力和履行的要求实时控制(快速反应)。控制电路采用模拟或数字电路可以实现单片机。在本文中,我们将介绍一种基于单片机PIC16F877单片机的直流电机速度控制系统的分类。 2.直流电机的调速原理 在图1中,电枢电压a U ,电枢电流a I ,电枢回路总电阻a R ,电机 常数a C 和励磁磁通Φ,根据KVL 方程,电机的转速为 Φ-= a a a a C R I U n a pN C 60= a a a a U R I U ≈- )1(63.0)(84.0)1()1()()1()(10--+-=--+-=k e k e k T k e a k e a k T k T d d d d i l T T Tf Kp a T T Kp a +==+ =10)1(
电子信息工程外文翻译 外文文献 英文文献
电子信息工程电路编程中的AT89C51单片机 译文标题电路编程中的AT89C51单片机 AT89C51 In-Circuit Programming 原文标题 作者Robert W.Sparks等译名国籍美国斯帕克等W.罗伯特 Atmel Corporation 原文出处 摘要本应用说明的是ATMEL公司AT89C51的电路可编程闪存的微控制器。为在电路可编程AT89C51的应用提出了与应用程序相关的例子,它的修改要求支持在线编程。这种方法显示在该应用程序中的AT89C51单片机可通过商业电话线远程改编。本应用笔记中描述的电路,仅支持5伏电压下编程,需要使用一个AT89C51-XX-5。标准A T89C51的需要12伏电压。该应用程序的软件可从ATMEL下载。 总论 当不在进行程序设计的时候,在电路设计中的AT89C51设计将变得透明化。 在编程期间必须重视EA/VPP这一脚。在不使用外部程序存储器的应用程序中,这脚可能会永久接到VCC。应用程序使用的外部程序存储器要求这一脚为低电平才能正常运行。 RST在编程期间必须为高电平。应该提供一种方法使得电路通入电源以后,使RST代替主要的复位电路起到复位的作用。 在编程过程中,PSEN必须保持低电平,在正常运行期间绝不能使用。ALE/ PRO在编程过程中输出低电平,在正常运行期间绝不能使用 在编程过程中AT89C5I / 端口是用于模式应用程序,地址和数据选择的,可能要该控制器从应用的电路隔离。如何做到这一点取决于应用程序 输入端 在编程过程中,控制器必须与应用电路的信号来源隔离。带有三个输出状态的缓冲区在应用程序之间插入电路和控制器,同时在编程时缓冲区输出三种状态。一个多路复用器用于信号源之间进行选择,适用于任何一方的应用电路或编程控制器电路的信号 输出端 如果应用的电路可以允许端口在编程过程中的状态变化,则不需要改变电路。如果应电路的状态,必须事先在编程过程中的保持不变,可能在控制器和应用电路中插入锁存。存在编程期间是可用的,并保存应用程序的电路状态 应用实 如所示应用是AT89C5一个移动的显示情况。此应用程序有在电路重新编程时结果以图表的形式显示的简单能力。文本显示被设计作为其硬件的一部分,不能在无改编况下变化 显示的文本可DI开关选择两种模式之一中进行。在第一种模式的时候,进入个字符从右边显示和快速移动,通过每个元素显示其在最后的装配位置的左侧。 第二个模式,信息在信息窗口中右到左移动显示。这种模式与常常在股票价格的显示所使用的方法类似 输出包括四DL14141段的积分解码器和驱动程序的字母数字显示器。这就生1名显示元素,每个数字0-的显示能力,是大写字母,标点符号和一些字符。可示字符ASCII码范围20H-5F上电复位电路和一6 MH的晶体振荡器完成应用件程序。无论外部程序存储器或外部数据存储器都时可用的
土木工程外文文献翻译
专业资料 学院: 专业:土木工程 姓名: 学号: 外文出处:Structural Systems to resist (用外文写) Lateral loads 附件:1.外文资料翻译译文;2.外文原文。
附件1:外文资料翻译译文 抗侧向荷载的结构体系 常用的结构体系 若已测出荷载量达数千万磅重,那么在高层建筑设计中就没有多少可以进行极其复杂的构思余地了。确实,较好的高层建筑普遍具有构思简单、表现明晰的特点。 这并不是说没有进行宏观构思的余地。实际上,正是因为有了这种宏观的构思,新奇的高层建筑体系才得以发展,可能更重要的是:几年以前才出现的一些新概念在今天的技术中已经变得平常了。 如果忽略一些与建筑材料密切相关的概念不谈,高层建筑里最为常用的结构体系便可分为如下几类: 1.抗弯矩框架。 2.支撑框架,包括偏心支撑框架。 3.剪力墙,包括钢板剪力墙。 4.筒中框架。 5.筒中筒结构。 6.核心交互结构。 7. 框格体系或束筒体系。 特别是由于最近趋向于更复杂的建筑形式,同时也需要增加刚度以抵抗几力和地震力,大多数高层建筑都具有由框架、支撑构架、剪力墙和相关体系相结合而构成的体系。而且,就较高的建筑物而言,大多数都是由交互式构件组成三维陈列。 将这些构件结合起来的方法正是高层建筑设计方法的本质。其结合方式需要在考虑环境、功能和费用后再发展,以便提供促使建筑发展达到新高度的有效结构。这并
不是说富于想象力的结构设计就能够创造出伟大建筑。正相反,有许多例优美的建筑仅得到结构工程师适当的支持就被创造出来了,然而,如果没有天赋甚厚的建筑师的创造力的指导,那么,得以发展的就只能是好的结构,并非是伟大的建筑。无论如何,要想创造出高层建筑真正非凡的设计,两者都需要最好的。 虽然在文献中通常可以见到有关这七种体系的全面性讨论,但是在这里还值得进一步讨论。设计方法的本质贯穿于整个讨论。设计方法的本质贯穿于整个讨论中。 抗弯矩框架 抗弯矩框架也许是低,中高度的建筑中常用的体系,它具有线性水平构件和垂直构件在接头处基本刚接之特点。这种框架用作独立的体系,或者和其他体系结合起来使用,以便提供所需要水平荷载抵抗力。对于较高的高层建筑,可能会发现该本系不宜作为独立体系,这是因为在侧向力的作用下难以调动足够的刚度。 我们可以利用STRESS,STRUDL 或者其他大量合适的计算机程序进行结构分析。所谓的门架法分析或悬臂法分析在当今的技术中无一席之地,由于柱梁节点固有柔性,并且由于初步设计应该力求突出体系的弱点,所以在初析中使用框架的中心距尺寸设计是司空惯的。当然,在设计的后期阶段,实际地评价结点的变形很有必要。 支撑框架 支撑框架实际上刚度比抗弯矩框架强,在高层建筑中也得到更广泛的应用。这种体系以其结点处铰接或则接的线性水平构件、垂直构件和斜撑构件而具特色,它通常与其他体系共同用于较高的建筑,并且作为一种独立的体系用在低、中高度的建筑中。
电动汽车电子技术中英文对照外文翻译文献
(文档含英文原文和中文翻译) 中英文资料外文翻译 原文: As the world energy crisis, and the war and the energy consumption of oil -- and are full of energy, in one day, someday it will disappear without a trace. Oil is not in resources. So in oil consumption must be clean before finding a replacement. With the development of science and technology the progress of
the society, people invented the electric car. Electric cars will become the most ideal of transportation. In the development of world each aspect is fruitful, especially with the automobile electronic technology and computer and rapid development of the information age. The electronic control technology in the car on a wide range of applications, the application of the electronic device, cars, and electronic technology not only to improve and enhance the quality and the traditional automobile electrical performance, but also improve the automobile fuel economy, performance, reliability and emissions purification. Widely used in automobile electronic products not only reduces the cost and reduce the complexity of the maintenance. From the fuel injection engine ignition devices, air control and emission control and fault diagnosis to the body auxiliary devices are generally used in electronic control technology, auto development mainly electromechanical integration. Widely used in automotive electronic control ignition system mainly electronic control fuel injection system, electronic control ignition system, electronic control automatic transmission, electronic control (ABS/ASR) control system,